Have you ever wondered how computers understand the different parts of speech in a sentence? If you have, then you’re in the right place! In this article, we’ll explore the concept of Part of Speech (POS) tagging and how it can be implemented using spaCy, a popular natural language processing (NLP) library.
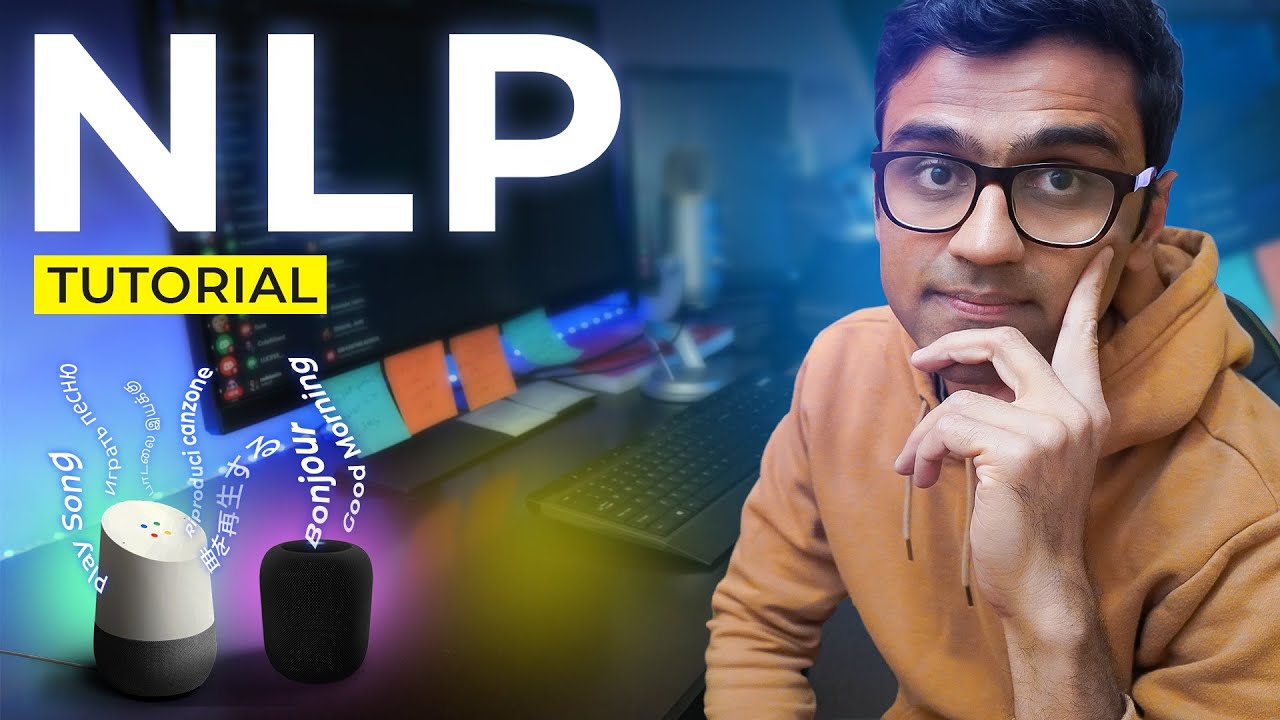
Contents
Understanding Part of Speech
Part of speech refers to the different grammatical categories that words can be classified into, based on their role and function in a sentence. For example, nouns, verbs, adjectives, adverbs, pronouns, and conjunctions are all parts of speech. Understanding these categories is fundamental to analyzing and understanding language.
When we look at a sentence like “Dhaval ate fruits,” we can break it down into different parts, which are called parts of speech. In this case, “Dhaval” is a noun, “ate” is a verb, and “fruits” is also a noun. Nouns represent people, places, things, or ideas, while verbs represent actions or states. This simple example demonstrates the basic concept of part of speech.
Let’s explore a few more examples to gain a better understanding.
In the sentence, “Elon would have been to Mars,” “Elon” and “Mars” are nouns, while “flew” is a verb. However, in the second sentence, we replace “Elon” with the pronoun “he.” Pronouns are words that substitute for nouns. Examples of pronouns include “he,” “she,” “our,” “you,” and “they.”
We can further enhance our sentence by adding adjectives, which describe nouns and add meaning to them. For instance, “I ate many fruits,” “I ate sweet fruits,” “I ate citrus fruits,” and “I ate tropical fruits” all utilize adjectives to provide additional context.
Adverbs, on the other hand, describe verbs, adjectives, or other adverbs. For example, “I slowly ate many fruits” and “I quickly ate many fruits” use adverbs to modify the verb “ate.”
These are just a few examples of the different parts of speech that exist in the English language. Overall, there are eight fundamental parts of speech, which include nouns, verbs, adjectives, adverbs, pronouns, conjunctions, prepositions, and interjections. Understanding these categories enables us to analyze and process language effectively.
Implementing Part of Speech Tagging with spaCy
Now that we have a basic understanding of part of speech, let’s see how we can implement POS tagging using spaCy.
First, we need to import the spaCy library and load the English model. We can then create a document using the sentence we want to analyze. By iterating through the tokens in the document and printing their part of speech, we can observe how spaCy assigns POS tags.
import spacy
# Load the English model
nlp = spacy.load('en_core_web_sm')
# Create a document with the sentence
doc = nlp("Dhaval ate fruits")
# Iterate through each token and print its part of speech
for token in doc:
print(token.text, token.pos_)
By running this code, we can see the part of speech assigned to each token in the sentence. Understanding the POS tags provided by spaCy allows us to gain deeper insights into the structure and composition of the sentence.
Enhancing POS Tagging with Additional Information
spaCy provides more than just the basic part of speech tags. We can utilize additional information such as tags and explanations to gain a more comprehensive understanding of the language.
For example, by accessing the .tag_
attribute of a token, we can obtain more detailed information about its part of speech. Additionally, using the spacy.explain()
function with the tag allows us to retrieve an explanation of the specific POS category.
import spacy
# Load the English model
nlp = spacy.load('en_core_web_sm')
# Create a document with the sentence
doc = nlp("Dhaval ate fruits")
# Iterate through each token and print its part of speech and explanation
for token in doc:
print(token.text, token.pos_, spacy.explain(token.tag_))
By adding these few lines of code, we can explore the specific tags assigned to each token in the sentence and gain a deeper understanding of their meanings.
Applying POS Tagging in Real-World Applications
POS tagging is a powerful technique that can be applied in various real-world applications. For example, we can use it to extract nouns or verbs from a text, count the occurrences of specific parts of speech, or even analyze the sentiment of a sentence by considering the POS of specific words.
To demonstrate this, let’s consider an example where we want to extract all the nouns and numbers from a given text. By filtering out unnecessary tokens such as spaces, punctuation marks, and other characters, we can focus solely on the relevant information.
import spacy
# Load the English model
nlp = spacy.load('en_core_web_sm')
# Create a document with the text
doc = nlp("I want to eat pizza, but I want to be healthy.")
# Filter out unnecessary tokens
filter_tokens = []
for token in doc:
if token.pos_ not in ['SPACE', 'PUNCT', 'X']:
filter_tokens.append(token)
# Count the occurrences of each POS category
pos_counts = {}
for token in filter_tokens:
pos = token.pos_
if pos in pos_counts:
pos_counts[pos] += 1
else:
pos_counts[pos] = 1
# Print the counts of each POS category
for pos, count in pos_counts.items():
print(pos, count)
In this example, we create a document with a given text and filter out tokens that are not relevant to our analysis. We then count the occurrences of each part of speech category and print the results. This approach allows us to perform various analyses based on the POS tags.
Conclusion
In this article, we explored the concept of Part of Speech (POS) tagging and how it can be implemented using spaCy. Understanding the different parts of speech enables us to analyze and process language effectively. By applying POS tagging in real-world applications, we can extract meaningful insights and enhance our understanding of text. So what are you waiting for? Start exploring the world of POS tagging and unlock the power of language analysis!
FAQs
Q: How does POS tagging help in NLP applications?
A: POS tagging plays a crucial role in natural language processing (NLP) applications. It allows us to extract meaningful insights from text by analyzing the different parts of speech. This information can be used to perform various tasks such as sentiment analysis, named entity recognition, information extraction, and more.
Q: Can POS tagging handle different languages?
A: Yes, POS tagging can be applied to different languages. However, the POS tag sets and rules may vary depending on the language. NLP libraries like spaCy provide models specifically trained for different languages, allowing us to perform POS tagging in multiple linguistic contexts.
Q: Are there any limitations to POS tagging?
A: Like any NLP technique, POS tagging has some limitations. It relies heavily on context and can sometimes be ambiguous, especially when dealing with words that can have multiple parts of speech. Additionally, POS tagging may not capture the semantic meaning of words but rather focuses on their grammatical role in a sentence.
Q: Are there any resources to learn more about POS and NLP?
A: Absolutely! There are various online resources, tutorials, and courses available to deepen your understanding of POS and NLP. You can start by exploring the official spaCy documentation, which provides comprehensive information about POS tagging and other NLP techniques. Additionally, there are numerous YouTube tutorials and online courses that cover POS and NLP in detail.
Remember, learning and experimenting with different NLP techniques is the key to mastering this exciting field!