NumPy is a powerful library that allows for efficient and fast mathematical operations on multi-dimensional arrays. In this article, we will explore the concept of broadcasting in NumPy and how it can be leveraged to perform complex computations with ease.
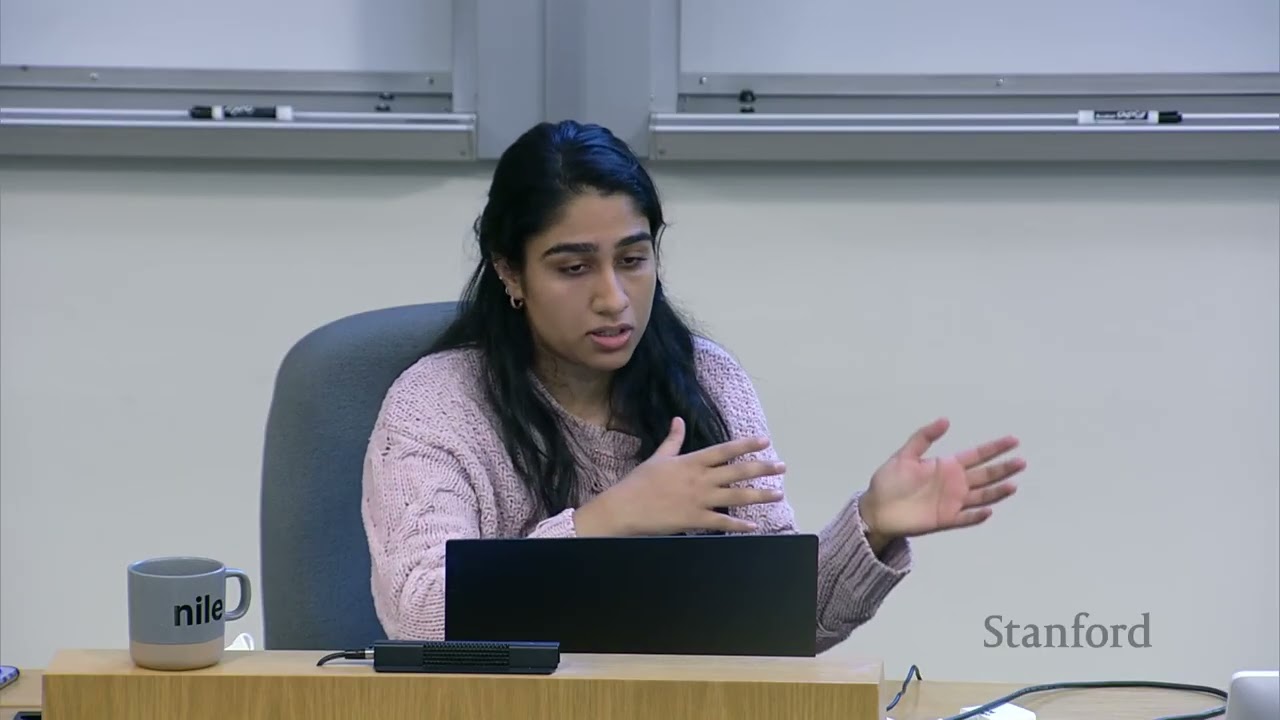
Contents
Introduction
NumPy is a Python library widely used for scientific computing and data analysis. It provides support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays efficiently. One of the key features of NumPy is its ability to perform broadcast operations, allowing for easy and efficient computations on arrays of different shapes.
Broadcasting in NumPy
Broadcasting is the term used in NumPy to describe the process of applying operations between arrays of different shapes. When performing a calculation between two arrays, NumPy automatically broadcasts the smaller array to match the shape of the larger array. This allows for element-wise operations to be performed directly, without the need for explicit loops.
Broadcasting Rules
To understand broadcasting better, let’s take a look at the broadcasting rules in NumPy:
- Rule 1: Arrays with different number of dimensions are broadcasted by adding singleton dimensions to the smaller array until their dimensions match.
- Rule 2: Arrays with dimensions of different lengths are broadcasted by adding singleton dimensions to the left of the smaller array until their dimensions match.
- Rule 3: Arrays with dimensions that differ by 1 are compatible and can be broadcasted. The array with the dimension of 1 is effectively stretched to match the shape of the other array.
Applying Broadcasting in NumPy
To illustrate the concept of broadcasting, let’s consider an example. Suppose we have an array x
of shape (3, 4) and we want to add a vector y
of shape (3, 1) to each row of x
. Instead of explicitly duplicating y
to match the shape of x
, we can use broadcasting to achieve the same result.
import numpy as np
x = np.array([[1, 2, 3, 4],
[5, 6, 7, 8],
[9, 10, 11, 12]])
y = np.array([[1],
[2],
[3]])
# Using broadcasting
result = x + y
print(result)
The output will be:
[[ 2 3 4 5]
[ 7 8 9 10]
[12 13 14 15]]
Here, we added y
to each row of x
using broadcasting. The shape of y
was automatically extended to match the shape of x
, making the operation possible.
Broadcasting enables us to perform complex operations on arrays of different shapes quickly and efficiently, eliminating the need for explicit loops and reducing code complexity.
Benefits of Broadcasting
Broadcasting offers several advantages in terms of code simplicity, readability, and computational efficiency:
- Simplified code: Broadcasting simplifies code by eliminating the need for explicit loops or duplicating arrays manually.
- Improved readability: Broadcasting allows for more concise and readable code, making it easier to understand and maintain.
- Enhanced computational efficiency: Broadcasting eliminates the need for unnecessary copies of data, making computations faster and more memory-efficient.
By leveraging the power of broadcasting in NumPy, you can write cleaner, more efficient code for performing complex computations on multi-dimensional arrays.
Conclusion
NumPy’s broadcasting capabilities provide a powerful and efficient way to perform computations on arrays of different shapes. By following the broadcasting rules and leveraging its benefits, you can simplify your code, improve readability, and enhance computational efficiency. Start utilizing broadcasting in your NumPy operations to unlock the full potential of this powerful library.
For more information about NumPy and its features, visit the official Techal website.
FAQs
Q: Can I perform broadcasting operations on arrays with different dimensions?
A: Yes, NumPy allows for broadcasting operations between arrays with different dimensions. The smaller array is broadcasted to match the shape of the larger array, enabling element-wise operations.
Q: Are there any limitations to broadcasting in NumPy?
A: Broadcasting works when the dimensions of the arrays are compatible. If the dimensions are not compatible, you may need to reshape the arrays or consider alternative approaches.
Q: How does broadcasting improve code efficiency?
A: Broadcasting eliminates the need for explicit loops and array duplication, reducing computational overhead and improving code efficiency. It allows for more concise and readable code, making it easier to understand and maintain.
Q: Can I broadcast operations across multiple dimensions?
A: Yes, broadcasting works across multiple dimensions. NumPy automatically matches the dimensions and performs element-wise operations, making complex computations on multi-dimensional arrays more manageable.
Q: Are there any performance considerations when using broadcasting in NumPy?
A: Broadcasting in NumPy is generally efficient and performs computations quickly. However, it’s important to be mindful of memory usage, particularly when working with large arrays or broadcasting across multiple dimensions.