Welcome back to another installment of our beginner C++ tutorial series. In this part, we will delve into the concepts of classes, objects, and member variables, and how they can help us enhance our game code. By the end of this tutorial, you will be able to keep track of the reticle position between frames, allowing you to freely move it across the screen. So let’s get started!
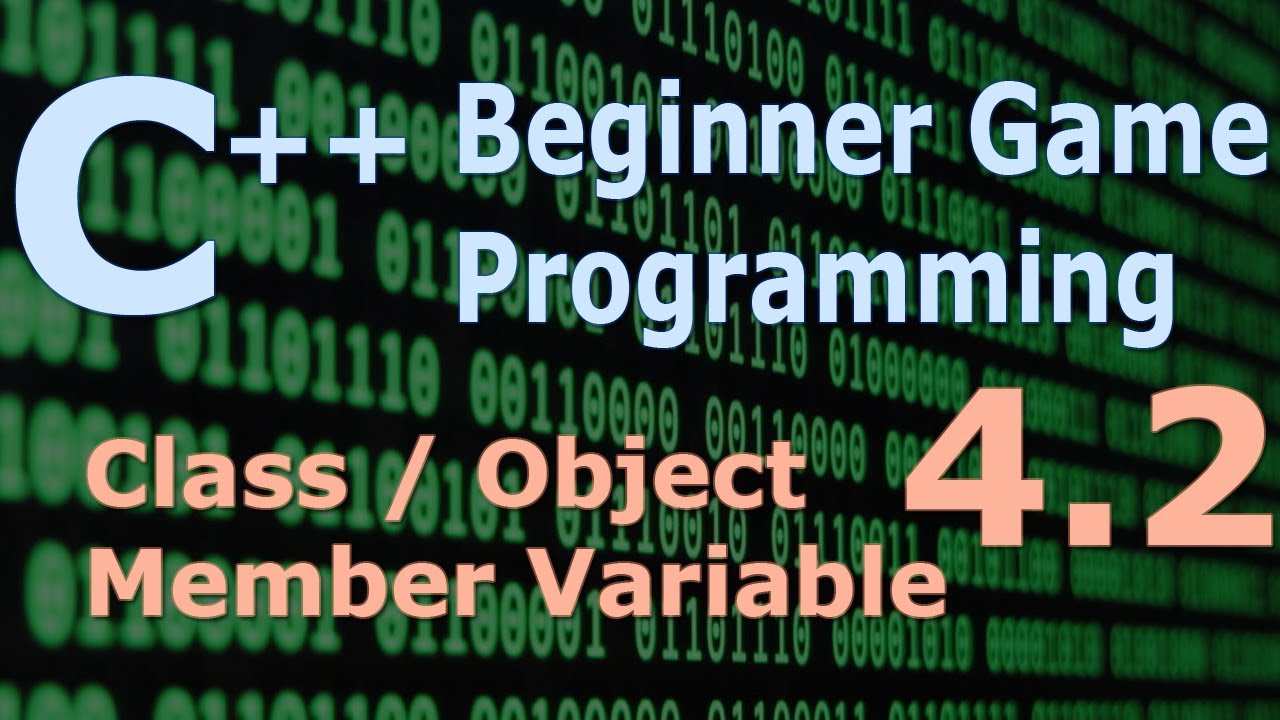
Contents
Classes and Objects – The Building Blocks of Object-Oriented Languages
Classes and objects are fundamental concepts in object-oriented programming languages like C++, Java, C#, and Python. They form the backbone of software systems in these languages.
Classes can be thought of as cookie cutters. Just as a cookie cutter determines the shape of the cookies made with it, a class defines the blueprint for creating one or more objects of that class type.
Objects, on the other hand, represent individual instances of a class. They are like the cookies created by using the cookie cutter. Objects are the entities that exist within a software system and can be manipulated or have operations performed on them.
In our game code, we have already been working with objects. For example, the graphics object represents the screen, and the window object contains the keyboard object, which allows us to check for pressed keys. These objects represent different aspects of our game system.
Member Variables – Tracking Object State
In addition to objects, classes also define member variables, which can be thought of as the physical parts or properties of an object. Member variables keep track of an object’s state or properties.
In our game, we want to update the reticle position between frames. To achieve this, we will move the reticle coordinates from the local composeFrame
function to the member variables of the game object.
To implement this, we need to modify the game.h
file. We define int x
and int y
as member variables for the game object, with initial values of 400 and 300, respectively.
class game {
public:
// other functions...
private:
int x = 400;
int y = 300;
};
With this change, the member variables x
and y
will now live for as long as the game object exists, rather than being local variables within the composeFrame
function. This allows us to access and modify their values between subsequent frame calls.
Next, we adjust the reticle movement speed by changing the increment per frame to 3 pixels. This will make the movement more controllable.
Now, when we run the program and use the arrow keys, we can move the reticle freely across the screen. We have successfully escaped the curse of losing the cursor position between frames!
Conclusion
In this tutorial, we explored the concepts of classes, objects, and member variables, and their role in object-oriented programming. By moving the reticle coordinates to member variables, we were able to retain their values between frames, allowing smooth and continuous movement.
Stay tuned for the next tutorial, where we will work with more practical examples and learn advanced techniques to control the reticle’s behavior. If you have any questions or feedback, leave a comment or visit our website Techal. Thanks for watching, and we hope you enjoyed the tutorial!