Welcome back to another hardware 3D tutorial! In today’s tutorial, we will explore the design and implementation of a keyboard component system using C++ and DirectX. This tutorial will provide you with all the necessary information to create a modular and efficient keyboard system for your software.
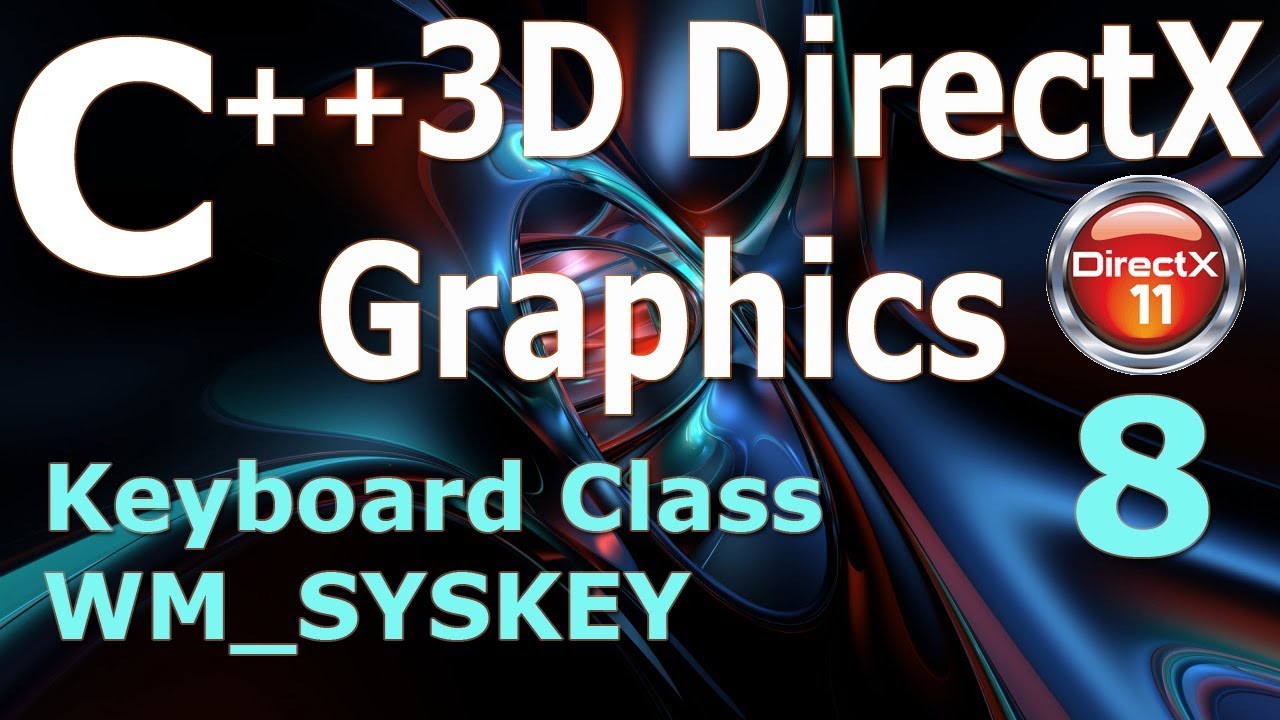
Contents
Designing the Keyboard Component
Before we dive into the code, let’s discuss the design of our keyboard component. Instead of creating one monolithic window class to handle all keyboard functionality, we will use a composition approach. This means we will break down the keyboard functionality into an individual keyboard class, which will be embedded in our window class.
Our keyboard component will consist of two main interfaces: the interface facing the window and the interface facing the client. The window-facing interface will handle incoming Windows messages and update the state of the keyboard object accordingly. The client-facing interface will allow the client software to access information about the keyboard input, such as the state of pressed keys and individual keyboard events.
To organize the data in our keyboard component, we will have two main types of data: the key state, which will hold the state of all the keys, and queues for key events and character events. The queues will store the events that are generated by keyboard input.
Implementing the Keyboard Component
Now let’s take a look at the code implementation of our keyboard component. We will create two files, keyboard.h
and keyboard.cpp
, to define and implement the keyboard class, respectively.
The keyboard.h
file defines the keyboard class and its interface. We have functions like keysPressed
to check if a key is currently being pressed, readKey
to pull a key event from the event queue, and keysEmpty
to check if there are any events in the queue. Similarly, we have functions for character events like readChar
, charsEmpty
, and clear
.
In the keyboard.cpp
file, we implement these functions and handle Windows messages like WM_KEYDOWN
and WM_KEYUP
to update the state of the keyboard object and populate the event queues. We also handle the WM_CHAR
message to handle character input. Additionally, we handle the WM_KILLFOCUS
message to clear the key state when the window loses focus.
Filtering Auto Repeat and System Keys
Auto repeat can be an issue when handling key events, especially in games. To address this, we check a specific bit in the lParam
of the Windows message to determine if the key is an auto repeat key. By selectively filtering auto repeat events, we can ensure smooth and accurate key input for games.
Another issue we encounter is handling system keys like the Alt key. We need to handle both WM_KEYDOWN
and WM_SYSKEYDOWN
messages to capture Alt key presses. By implementing both message handlers and using a fall-through behavior, we can handle system keys and regular key presses in the same code.
Testing the Keyboard Component
To test our keyboard component, we can add a simple functionality to display a message box when the spacebar is pressed. By checking the state of the keyboard object in the message pump, we can determine if the spacebar is currently being pressed and trigger the message box accordingly.
Conclusion
In this tutorial, we have explored the design and implementation of a keyboard component system using C++ and DirectX. We have learned how to handle key events, filter auto repeat, and capture system keys like the Alt key. By following these steps, you can create a modular and efficient keyboard component for your software.
Thank you for reading this comprehensive guide. If you enjoyed this article, please consider sharing it with your friends and colleagues. Stay tuned for more tutorials on hardware 3D programming!
FAQs
-
Q: Can I use the keyboard component for text input?
A: Yes, the keyboard component can handle text input by capturing character events. You can use thereadChar
function to pull characters off the event queue. -
Q: How can I clear the event queues?
A: You can use theclear
function to clear both the key event queue and the character event queue. -
Q: Is it possible to disable auto repeat for specific keys?
A: Yes, you can selectively filter auto repeat events by checking the auto repeat bit in the Windows message. This allows you to control which keys trigger auto repeat.
Conclusion
In this article, we have discussed the design and implementation of a keyboard component system using C++ and DirectX. By following this guide, you can create a modular and efficient keyboard system for your software. If you want to learn more about technology and explore other informative articles, visit Techal.
Thank you for reading, and stay tuned for more exciting technology content from Techal!