Welcome back to another exciting episode of Beginner C++ Tutorial 3! Today, we are diving into the world of control flow in programming. We will be exploring the if statement, which allows us to make decisions in our programs, as well as the use of boolean variables. We will also touch on keyboard input. So, let’s dive right in!
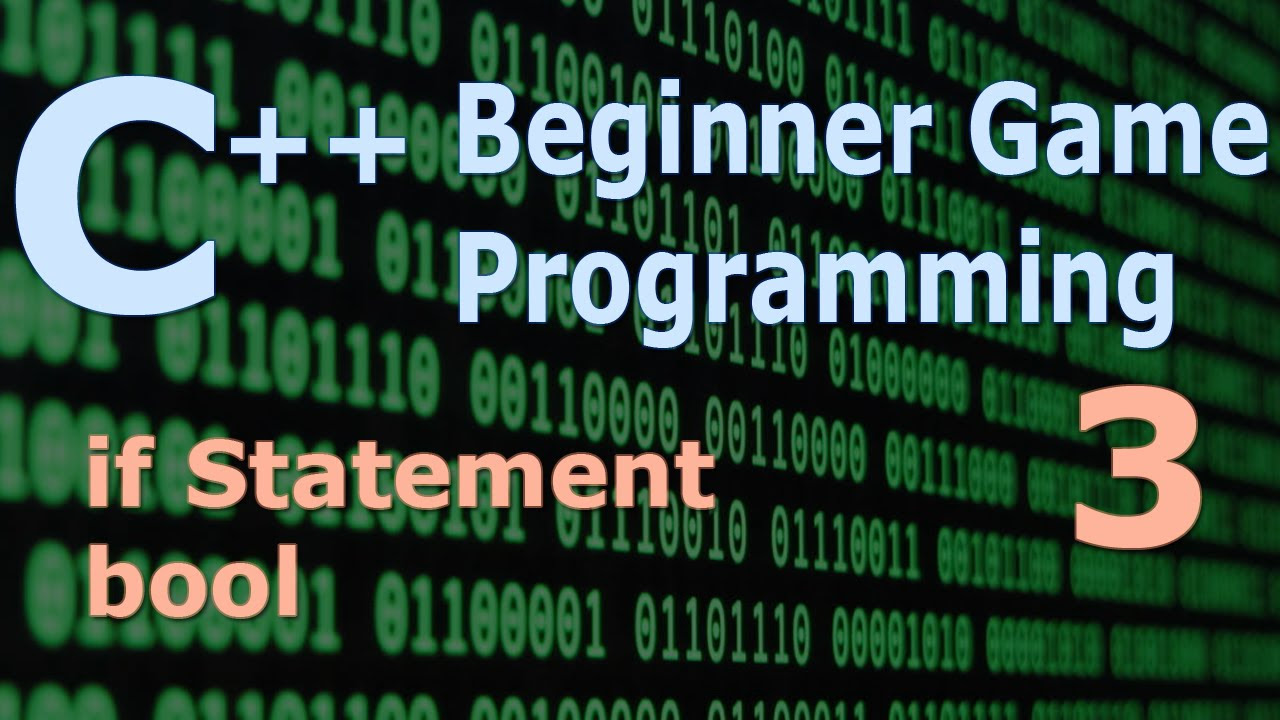
Contents
The Power of the if Statement
At its core, the if statement is a powerful tool that allows us to execute code based on whether a certain condition is true or false. It is a way of branching our program’s logic. Picture it like a fork in the road, where we choose a path based on a condition.
To illustrate this, let’s start with a simple example.
if (condition) {
// Code to be executed if the condition is true
}
In this example, the condition
can be any expression that evaluates to either true or false. If the condition is true, the code block inside the curly braces will be executed. If the condition is false, the code block will be skipped.
To make this concept more concrete, let’s consider a real code example. Imagine we have a game where we want to move a reticle on the screen based on user input. We can use the if statement and boolean variables to achieve this.
bool isUpKeyPressed = window.keyboard.keyIsPressed(VK_UP);
if (isUpKeyPressed) {
// Code to move the reticle up
}
In this example, we use the keyIsPressed()
function from the keyboard
object (which is a member of the window
object) to check if the up arrow key is being pressed. If it is, the code inside the if statement block will be executed, moving the reticle up.
Nested if Statements
We can also nest if statements to create more complex branching logic. This allows us to handle multiple conditions in a specific order. Each if statement will be evaluated sequentially, and only the code block associated with the first true condition will be executed.
if (condition1) {
// Code to be executed if condition1 is true
} else if (condition2) {
// Code to be executed if condition1 is false and condition2 is true
} else {
// Code to be executed if both condition1 and condition2 are false
}
Here’s an example to illustrate this:
if (isUpKeyPressed) {
// Code to move the reticle up
} else if (isDownKeyPressed) {
// Code to move the reticle down
} else {
// Default code when no movement keys are pressed
}
In this example, if the up arrow key is pressed, the reticle will move up. If the up arrow key is not pressed, but the down arrow key is pressed, the reticle will move down. And if neither key is pressed, the default code will execute.
Bringing it All Together: Homework Assignment
Now it’s time for some hands-on practice! Your homework assignment is to create a program that allows you to control the position, color, and shape of a cursor on the screen using keyboard input.
Here are the requirements for your program:
- Use the arrow keys to control the position of the cursor within a grid of nine positions.
- Use the control key to change the color of the cursor when pressed.
- Use the shift key to change the shape of the cursor when pressed.
- Allow simultaneous changes of color and shape by holding down both the control and shift keys.
- Be creative with the shape of the cursor, but keep it appropriate for all audiences.
Remember to have fun with this assignment and explore the possibilities of what you can create using control flow and keyboard input!
FAQs
Q: How does the if statement work?
A: The if statement allows you to execute code based on the truthfulness of a condition. If the condition is true, the code block associated with the if statement will be executed. If the condition is false, the code block will be skipped.
Q: Can I use multiple conditions with the if statement?
A: Yes! You can use the else if clause to chain multiple conditions together. This allows you to create branching logic based on different conditions.
Q: Can I nest if statements?
A: Absolutely! You can nest if statements inside each other to create more complex branching logic. Just make sure to pay attention to the order in which the conditions are checked, as only the code block associated with the first true condition will be executed.
Conclusion
In this tutorial, we explored the power of the if statement and how it allows us to make decisions in our programs. By combining the if statement with boolean variables and keyboard input, we can create interactive programs that respond to user input.
Don’t worry if you don’t fully grasp every concept just yet. We will continue practicing with these topics in future tutorials. Remember, the journey to becoming a skilled programmer is all about building upon your knowledge one step at a time.
Stay tuned for the next tutorial, where we will further explore the magic behind moving the reticle on the screen. Until then, happy coding!