Welcome back to Advanced C++ Tutorial 2.2! In the previous video, we learned about translation scaling, screen coordinate transformation, and entity setup. Now, we’re going to delve into implementing camera scrolling, which is an essential aspect of game development. So, let’s get started!
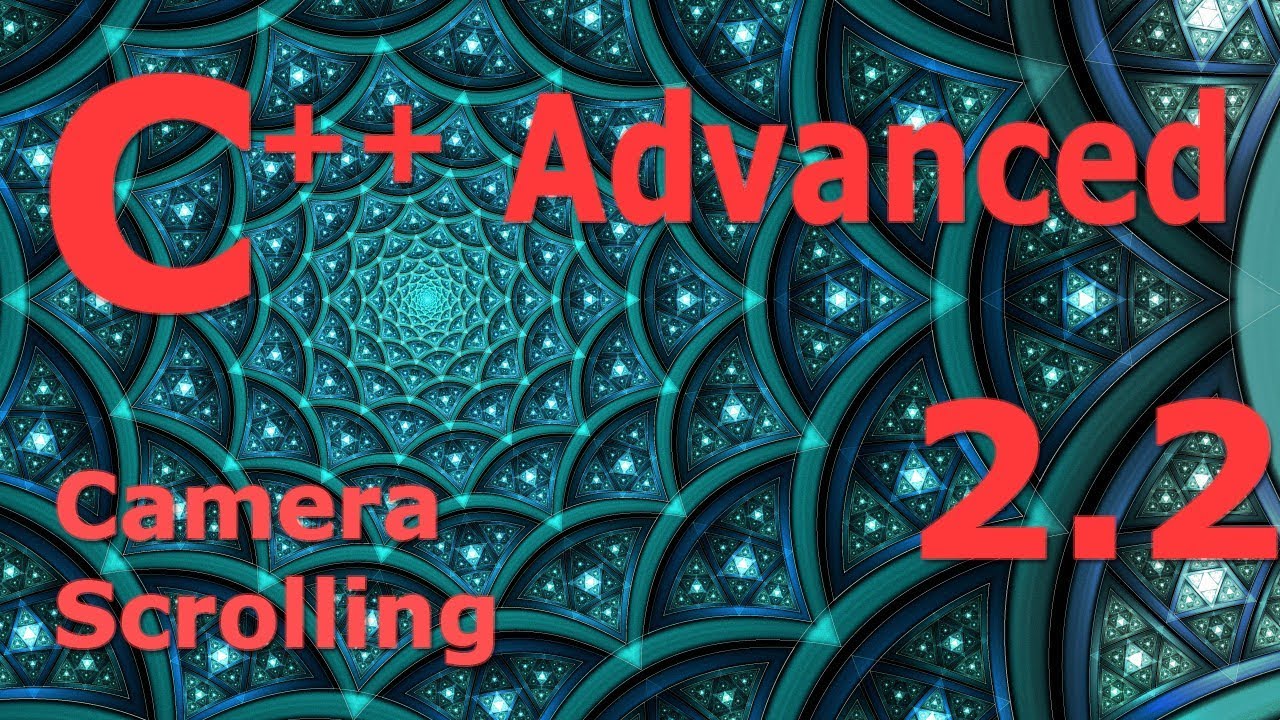
Contents
Scrolling with a Camera System
In real games, the game world is often too large to fit entirely on the screen. This is where camera scrolling comes in. Scrolling allows us to follow the action and view different parts of the world, usually by tracking the player’s character. Now, let’s understand how this works mathematically and in terms of transformations.
When we scroll the camera down, the scene appears to move up on the screen. Similarly, when we scroll the camera up, the scene appears to move down. In other words, scrolling the camera down is equivalent to scrolling the entire world up. To achieve this effect, we need to apply a translation to all the objects in the world, moving them up or down depending on the camera movement.
Implementing the Camera
To implement camera scrolling, we need a camera component in our pipeline. The camera component holds the position of the current view of the world and applies a translation to all the objects. Let’s start by creating a camera class that stores the camera’s position and a reference to the coordinate transformer.
class Camera {
private:
Vector2 position;
CoordinateTransformer& coordinateTransformer;
public:
Camera(const Vector2& initialPosition, CoordinateTransformer& ct)
: position(initialPosition), coordinateTransformer(ct)
{}
// Getter and setter methods for the camera's position
void moveBy(const Vector2& offset) {
position += offset;
}
void moveTo(const Vector2& newPosition) {
position = newPosition;
}
void translate(const Vector2& offset) {
position += offset;
coordinateTransformer.drawClosedPolygonPolyline(offset);
}
};
In the camera class, we have methods to move the camera by an offset or to a specific position. The translate
method applies the camera translation to the coordinate transformer, which passes it down the pipeline.
Now, let’s implement the camera in the game code. We’ll add a camera to the game, initialize it with the coordinate transformer, and use it to draw all the stars in the scene.
class Game {
private:
std::vector<Entity> scene;
Camera camera;
public:
// ...
void composeFrame() {
for (const auto& entity : scene) {
camera.draw(entity.getDrawable());
}
}
};
By calling the draw
method of the camera, we can pass the drawable object of each entity to the coordinate transformer for rendering. This way, the camera translation is applied to all the objects in the scene.
Zooming with the Camera
In addition to scrolling, we can also implement zooming with the camera. When we zoom in, objects appear larger on the screen, and when we zoom out, objects appear smaller. Zooming is essentially a scaling operation, and we can extend our camera class to include scaling capability.
class Camera {
// ...
private:
float scale;
public:
// ...
void zoom(float scaleAmount) {
scale *= scaleAmount;
}
};
In the zoom
method, we multiply the current scale by the scale factor to zoom in or zoom out. We can apply this scaling to the coordinate transformer, allowing us to zoom in or out of the scene.
Combining Transformations
To optimize our transformations, we can combine multiple translations into a single transformation and apply it once to all the vertices. This reduces the number of operations needed for each vertex and improves performance. However, it’s important to consider the order of transformations.
For example, scaling should be applied before translation if we want to scale objects around their center. If we apply translation before scaling, the center of the object will be displaced during scaling.
To combine translations, we can use a Drawable
object that stores the model, translation, and scale transformations. Then, in the rendering step, we can apply the transformations to all the vertices at once. This approach improves efficiency and reduces the need for multiple transformation loops.
Homework Tasks
For your homework, I have a series of tasks to help you further explore and practice the concepts we covered:
-
Generate a scene with a large number of randomly placed stars. Each star should have a random size and shape, creating a realistic star field. Ensure that the stars do not overlap.
-
Implement click-and-drag scrolling for the camera component. This will allow the player to scroll through the scene by clicking and dragging the screen.
-
Modify the
Drawable
class to store a reference to the polyline instead of making a copy. This optimizes memory usage and reduces the need for duplicating data. -
Improve performance by only drawing the stars that are within the visible screen region. Implement a mechanism to skip drawing the stars outside the visible area, saving computational resources.
-
For added visual appeal, animate the stars by making them pulse in size and change color rhythmically. This will bring your scene to life and make it more engaging.
Completing these tasks will enhance your understanding of transformations, pipelines, and various optimization techniques. Remember, the design pattern presented in this tutorial is just one approach, and there are many other ways to achieve similar results.
Thank you for watching! If you found this tutorial helpful, please hit the like button and stay tuned for more advanced C++ topics. Happy coding, and see you soon!
FAQs
-
How do I implement click-and-drag scrolling for the camera?
- You can capture mouse events (mouse button down, move, and release) and update the camera’s position based on the mouse movement. Translate the camera by the mouse offset to achieve the scrolling effect.
-
How do I animate the stars to pulse in size and change color?
- Modify the rendering logic to apply the animation transformations to the stars. You can use scaling and color shading algorithms to achieve the desired effect. Experiment with different techniques and parameters to create visually appealing animations.
Conclusion
In this tutorial, we explored the implementation of camera scrolling in C++. We learned how to use the camera component to scroll through a game world, apply translations and scaling, and optimize transformations by combining them into a single step. Completing the homework tasks will help solidify your understanding of these concepts. Stay tuned for more advanced C++ tutorials, and happy coding!
For more informative articles and exciting technology updates, visit Techal.