Welcome back to another lesson in our Beginner C++ DirectX Game Programming series! In this lesson, we will be implementing some key features in our game. Let’s get started!
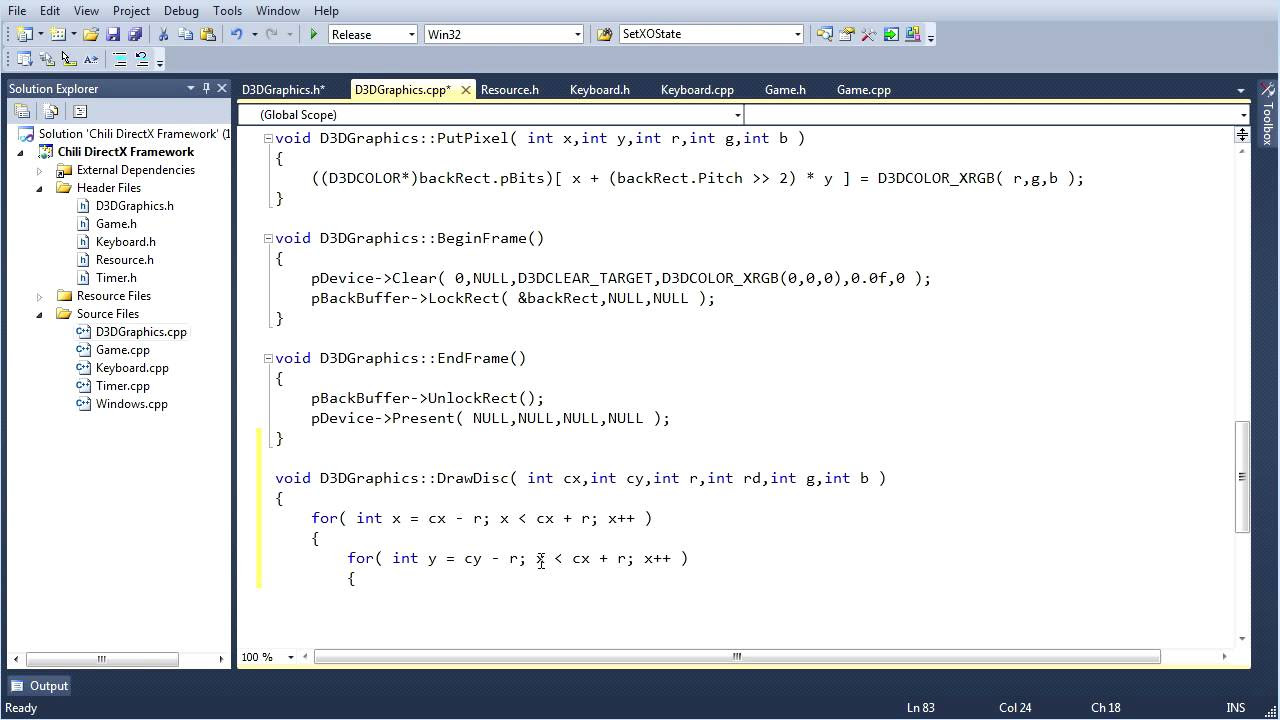
Adding Random Movement to Poo
One of the features we want to add is random movement to the poo objects in our game. Currently, the poos move in a straight line without any variation. To add some randomness to their movement, we can introduce a chance for each poo to change its velocity.
To do this, we’ll start by creating a local variable called lottery
to generate a random number between 0 and 200. We’ll use this number to determine which poo object will have its velocity changed.
Next, inside our update loop for the poos, we’ll check if the current poo’s index matches the lottery
number. If it does, we will randomize its velocity using the existing code. This way, every frame, there is a chance for one poo to have its velocity changed.
Implementing Collision Detection with the Goal
Another feature we want to add is collision detection with the goal. Currently, when we collect the goal, nothing happens. We want the game to respond when the face collides with the goal.
To achieve this, we’ll modify our game logic to check if the distance between the face and the goal is less than the sum of their radii. If it is, we can consider the goal collected. We’ll reset the goal to a new position and increase the number of poos in the game.
To implement this, we’ll modify the update loop for the face and add the collision detection logic after updating the position. If the distance between the face and the goal is smaller than the sum of their radii, we reset the goal and increase the number of poos.
Summary
In this lesson, we implemented two key features in our game: random movement for the poo objects and collision detection with the goal. We added the ability for poos to change their velocity randomly, creating a more dynamic gameplay experience. We also implemented collision detection between the face and the goal, allowing the player to collect the goal and increase the number of poos in the game.
In the next lesson, we will learn about structures and how they can be used to organize and manage our game data more efficiently. Stay tuned for more exciting lessons in our Beginner C++ DirectX Game Programming series!