Python, known for its simplicity and ease of learning, is often criticized for being relatively slow compared to compiled languages. This is mainly due to the fact that Python is an interpreted language with dynamic typing and a global interpreter lock. However, there are effective methods to speed up Python code, particularly when it comes to intensive tasks like calculating the Mandelbrot fractal.
In this article, we will explore step-by-step ways to accelerate Python code using parallel computing on both the processor and graphics chip. To demonstrate these methods, we will focus on creating a program that allows users to traverse the Mandelbrot set.
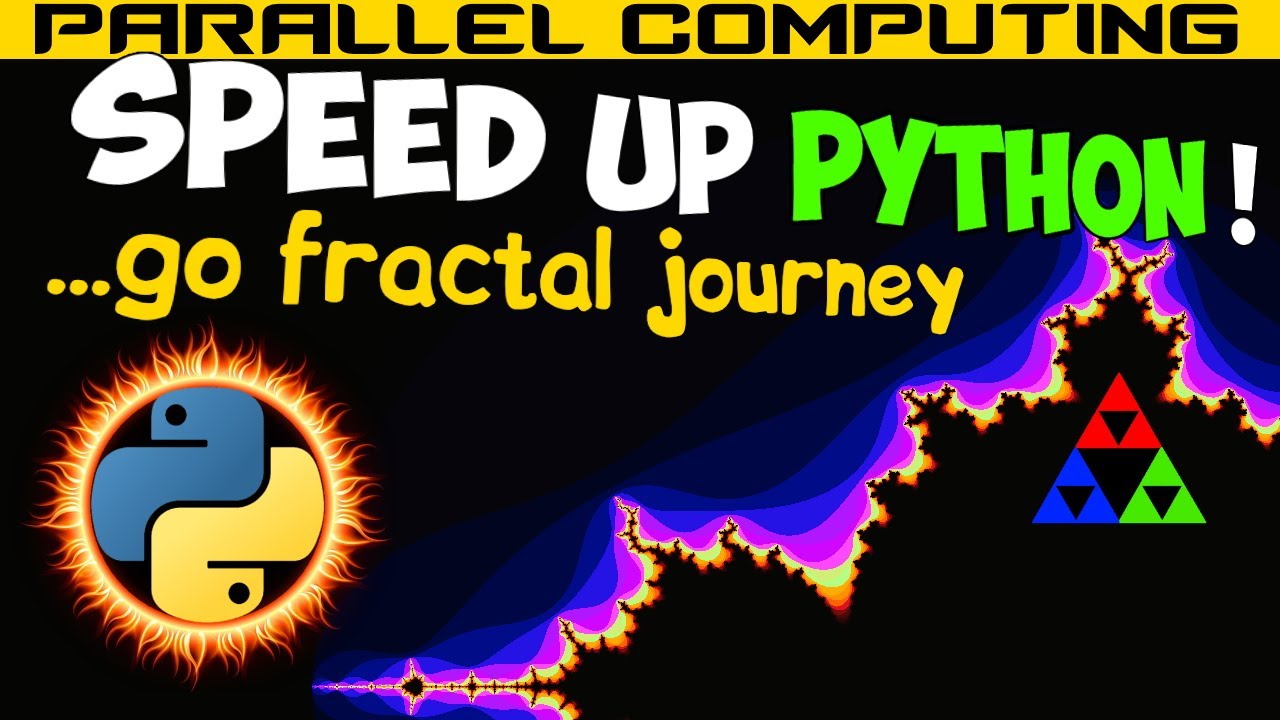
Contents
Choosing the Framework: Pygame
Before we delve into the optimization techniques, let’s first decide on the framework for our application. Since we plan to create an interactive program, we will use the Pygame graphics library to display the Mandelbrot fractal. Pygame provides a simple and intuitive interface for working with graphics in Python.
The Mandelbrot Fractal
To understand the optimizations, it’s important to have a basic understanding of the Mandelbrot fractal. The Mandelbrot set is constructed based on a mathematical relation where each complex number is formed by adding the square of the previous number to a constant value, denoted as “c”. The set is bounded within a circle with a radius of 2, meaning the modulus of the number “z” should not exceed this value.
To display the Mandelbrot fractal, we iterate through each coordinate on the plane, calculate the corresponding complex number “c”, and determine how many iterations are needed for the modulus of “z” to exceed 2. This iteration count can then be mapped to a grayscale or color value, allowing us to visualize the fractal.
Starting with Low Resolution
To begin, we start with a low resolution image of the Mandelbrot fractal. By selecting a smaller window size, we can reduce the initial computational load. Additionally, we implement a scaled mode that allows us to scale the image to the current resolution of the screen.
We encapsulate the main functionalities within a “Fractal” class, which includes creating a numpy array as our canvas for drawing, methods for rendering and updating the state, and a “run” method to launch the program. An instance of the “Fractal” class is created in the application, and the “run” method is called in the main loop.
Using Pure Python
Initially, when using pure Python, the performance is quite low, averaging around 5 frames per second. However, by increasing the image resolution and applying a gradient texture to the fractal, we can make it visually more appealing. Unfortunately, the number of frames drops significantly.
Leveraging Numpy
To improve performance, we can make use of the numpy library, which provides fast and efficient numerical operations in Python. By utilizing vectorized operations on numpy arrays, we can eliminate the need to iterate over coordinates and significantly increase the speed of our code.
With numpy, the performance increases dramatically. By excluding coordinate iteration and using vectorized operations, we achieve an astonishing four times increase in frames per second, reaching a level of over 20 frames per second.
Optimizing with Numba
Another optimization technique involves using Numba, a just-in-time (JIT) compiler that translates Python code into fast machine code. By applying the @jit decorator with specific parameters, we can optimize our code further.
By getting rid of the square root calculation and replacing it with a comparison of the sum of squares, we achieve an even greater performance boost. With Numba, we see an increase in frames per second by 260 times compared to the original code.
Parallel Computing with Numba
Numba also provides the ability to leverage parallel computing. By activating the parallel parameter and replacing the range function with the p range generator, we can take advantage of parallel computation.
Enabling parallel computing results in an additional performance increase of almost 50%, compared to regular Numba. In total, we achieve a staggering 360 times speedup compared to the original code.
Tai Chi: High-Performance Computing
Tai Chi is another JIT compiler specifically designed for high-performance calculations, utilizing parallel computing on both processors and graphics chips.
By using the tai chi module, we can further optimize our code. However, in Tai Chi, there is no data type specifically for complex numbers. Instead, we represent complex numbers as two-dimensional vectors, with the real part in the first component and the imaginary part in the second component.
Using the functionalities of the Tai Chi module, we experience a significant increase in performance. By leveraging parallel computing, we achieve a 400 times speedup compared to the original code.
Considering Hardware Limitations
It’s important to note that the performance results may vary depending on your hardware. In the case of this article, the performance was affected by the hardware used, particularly the graphics built into the processor. Therefore, the results largely depend on the specific hardware configuration.
Conclusion
In conclusion, there are numerous ways to speed up Python code, especially when dealing with computationally intensive tasks like calculating the Mandelbrot fractal. By leveraging frameworks like Pygame, libraries like numpy, and JIT compilers like Numba and Tai Chi, we can achieve significant performance improvements.
Although hardware limitations may affect the actual speedup achieved, the overall results indicate that optimizing Python code can lead to substantial performance gains. So go ahead and explore these techniques to enhance the speed of your Python applications and unleash the full potential of the language.
FAQs
Q: Are these optimization techniques limited to the Mandelbrot fractal?
A: No, these techniques can be applied to various areas of Python programming where performance improvements are desired. The Mandelbrot fractal serves as a practical example to showcase the effectiveness of these optimizations.
Q: Can these optimization techniques be used with other programming languages?
A: While some techniques, like leveraging numpy, can be applied to other programming languages that support similar libraries, others, like Numba and Tai Chi, are specific to Python. However, similar performance optimization principles can be applied in other languages with their respective tools and frameworks.
Q: Where can I learn more about the Tai Chi module?
A: For more information about the Tai Chi module and how to use it for high-performance computing, you can visit the official Tai Chi website.
Conclusion
In summary, speeding up Python code is crucial for optimizing performance in computational tasks. By utilizing libraries like numpy and JIT compilers like Numba and Tai Chi, we can significantly increase the speed of Python programs. Whether you’re working with the Mandelbrot fractal or any other Python project, these optimization techniques can help unleash the full potential of your code. So go ahead, explore, and accelerate your Python journey.