In the world of programming, two key concepts often come into play: composition and inheritance. These concepts are especially relevant when working with object-oriented languages like C++. Understanding how to use both composition and inheritance effectively can greatly enhance your code and improve your overall design.
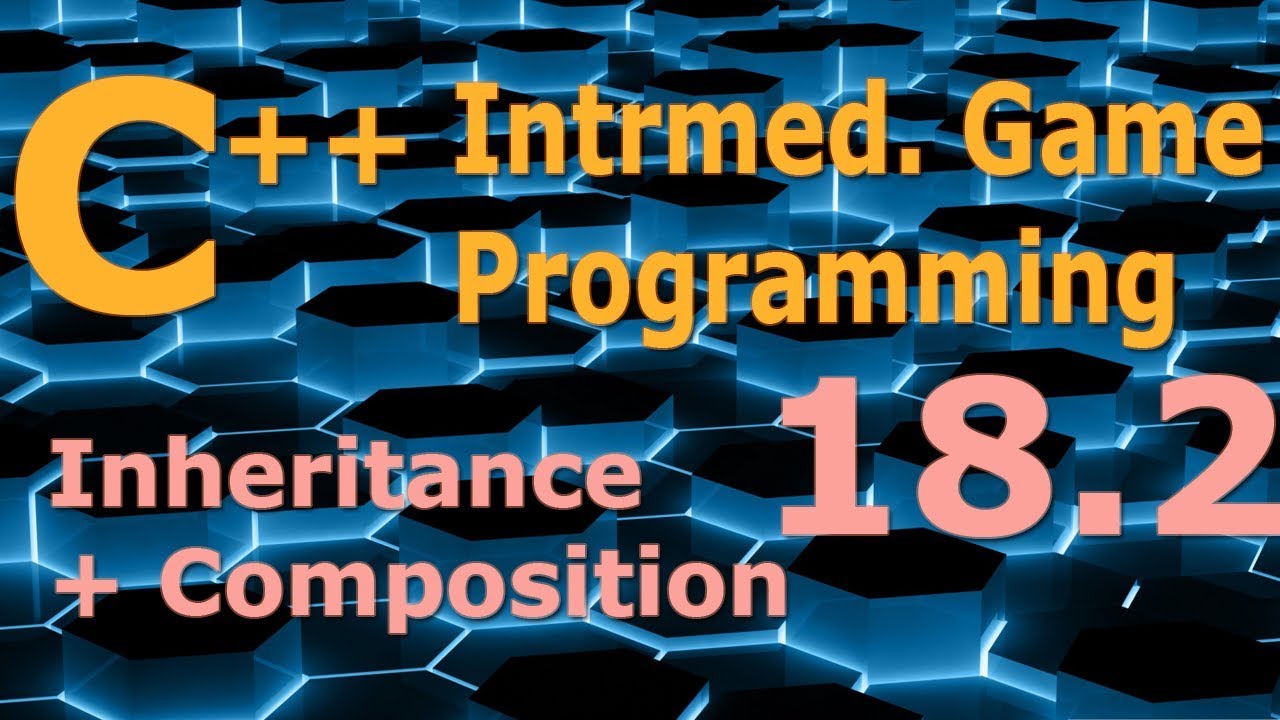
Contents
The Problem with Inheritance Alone
In this article, we will explore a common mistake many programmers make: relying solely on inheritance to solve a problem. While inheritance can be a powerful tool, it is essential to recognize its limitations and consider alternative approaches.
Consider a scenario where we have fighters in a game. Each fighter has a specific attack, but we want to introduce different attacks based on the weapon they wield. One possible solution is to use inheritance to create separate classes for each fighter and their corresponding weapon. However, this approach quickly becomes unmanageable.
For instance, if we have 10 types of weapons and 10 types of fighters, we would end up with 100 different concrete fighter classes. Maintaining and updating these classes would be a nightmare. Additionally, implementing unique special abilities for each fighter would result in redundant code.
The Power of Composition and Inheritance Together
To address these challenges, we can combine composition and inheritance to create a more flexible and scalable solution. Instead of creating separate classes for each fighter and their weapon, we can use composition to associate a weapon with a fighter.
In this approach, the fighter class becomes an abstract class that holds a pointer to a weapon object. The weapon class can be inherited to create different types of weapons, each with its own behavior. The fighter class then accesses the weapon’s functionalities through the pointer, allowing for dynamic dispatch and polymorphism.
This composition and inheritance combination eliminates the need for an excessive number of concrete fighter classes. Instead, we have a more manageable number of concrete classes that represent different combinations of fighters and weapons. This simplifies maintenance and prevents code duplication.
Performance Considerations
One concern often raised when using virtual functions, a common feature of polymorphism, is the performance impact. While it is true that virtual functions introduce overhead due to pointer manipulation and function lookups, this impact is generally negligible.
In most cases, the performance difference between using virtual functions and other optimization techniques is insignificant. Premature optimization based on assumptions about performance impacts can lead to overly complex and less maintainable code. It is essential to focus on code clarity and maintainability while considering performance optimizations only when necessary.
Conclusion
To summarize, a combination of composition and inheritance can greatly enhance the design and flexibility of your code. By leveraging these concepts effectively, you can create maintainable and scalable solutions.
In scenarios where a large number of concrete classes would be required, composition and inheritance allow for more manageable code structures. Additionally, concerns about performance impacts should be balanced with the advantages of code clarity and maintainability.
By understanding when and how to use composition and inheritance, you can develop more robust and efficient code in C++. Embrace these concepts, experiment with different design patterns, and continue to refine your code to achieve the best results.