Welcome back to the second lecture of our introductory series. In this lecture, we will continue our discussion on matrices and vectors, and delve deeper into the logic structures used in coding. These concepts are fundamental to analyzing and manipulating data in programming.
When you open MATLAB, you will see two windows: the command window and the editor window. The command window allows you to type commands and evaluate them, while the editor window is where you can write longer pieces of logic that can be executed all at once.
To review from last time, let’s consider an example. Suppose we want to create a variable named “a” that contains the numbers from 1 to 10. We can achieve this by typing:
a = 1:10;
By suppressing the output with a semicolon at the end, we load the variable “a” into the workspace. If we want to see the content of “a,” we can simply type “a” in the command window.
It’s always useful to check the size of the vectors or matrices we create. To do this, we use the size
function. For example, typing size(a)
will show that “a” has one row and 10 columns. If we only want to know the number of rows or columns, we can use the size
function with the appropriate input, such as size(a, 1)
for the number of rows or size(a, 2)
for the number of columns.
We can access specific elements inside a vector using parentheses. For example, if we want the first element of “a,” we can write a(1)
. Similarly, if we want the ninth element, we can write a(9)
. Additionally, we can use variables to access elements. For instance, if we set i = 9
, we can write a(i)
to access the ninth element of “a.”
Now, let’s move on to discussing conditionals. Conditionals allow us to ask questions that can be answered with a “true” or “false.” In MATLAB, we use double equal signs (==
) for equality, less than or equal to (<=
), greater than or equal to (>=
), greater than (>
), and less than (<
). To check for inequality, we use the exclamation mark followed by an equal sign (!=
).
For example, if we want to check if one is equal to one, we can write 1 == 1
. The output will be 1, indicating that it is true. If we compare 1 to 2, the output will be 0, indicating that it is false.
Conditionals can be combined with variables and other logical operations. For example, we can write a(5) > 100
to check if the fifth element of “a” is greater than 100. The result will be true if the condition is satisfied.
Building on conditionals, we can use the if-else structure to execute different sets of instructions based on the outcome of a conditional statement. Suppose we want to display the word “hooray” if the fifth element of “a” is greater than 105. We can write the following code:
if a(5) > 105
disp('hooray');
else
disp('boo');
end
If the condition is true, the code will display “hooray.” Otherwise, it will display “boo.”
The if-else structure provides a way to handle different scenarios in programming, depending on the conditions met. It is important to close the if statement with an end
keyword.
To summarize, we have covered the basics of matrices, vectors, conditionals, and the if-else structure in MATLAB. These concepts form the building blocks of logical thinking in coding. By using variables, conditionals, and control structures like if-else, we can create powerful programs that make decisions based on specific conditions.
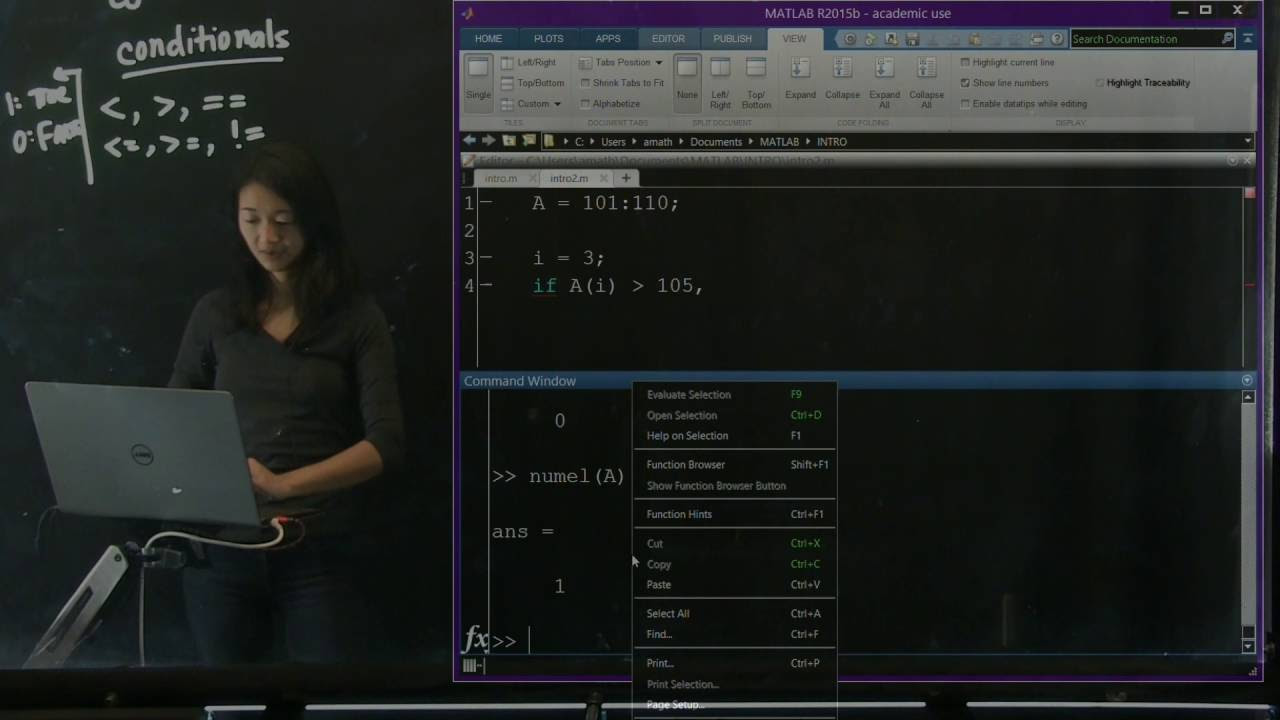
Contents
FAQs
Q: Can I use the if-else structure for more complex conditions?
A: Definitely! You can combine multiple conditionals using logical operators, such as &&
(logical AND) and ||
(logical OR), to create complex conditions in the if-else structure.
Q: How can I execute multiple lines of code inside the if statement?
A: You can simply write the desired code inside the if statement. Make sure to indent it properly for readability. The code inside the if statement will be executed if the condition is true.
Conclusion
In this article, we have explored the basics of loops and logic in MATLAB. We have learned how to create vectors and matrices, access their elements, and use conditionals to make logical comparisons. Additionally, we have seen how the if-else structure allows us to execute different instructions based on the outcome of a condition. By mastering these concepts, you can start building more advanced programs that perform complex logical operations.
For more information on MATLAB and its features, visit the official Techal website.