Welcome back to the Ray Tracing series! In this episode, we are going to delve into the topic of rendering multiple objects in our scenes. We will explore how to structure our scenes and discuss the theory behind rendering multiple objects.
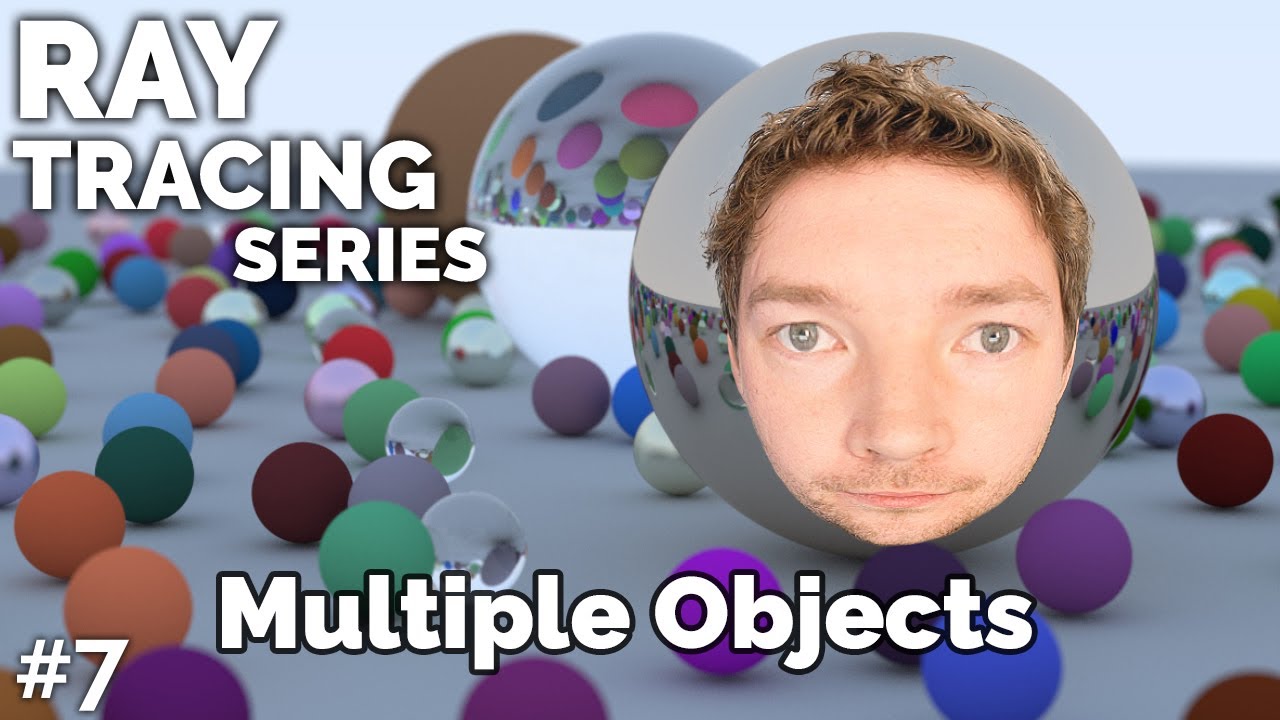
Contents
Understanding Multiple Objects
Before we dive into the technical details, let’s take a moment to understand the concept of rendering multiple objects. In our current viewport, we have a camera that shoots rays from a specific origin and direction. We also have a mathematical description of a single sphere. For each ray, we check if it intersects the sphere and calculate the hit position, normal, and color.
To render multiple objects, we need to extend this process. We need to perform the ray-sphere intersection test for each sphere in the scene. If a ray intersects multiple spheres, we choose the closest one to the camera for evaluation. If none of the spheres are hit, we return the background color.
Implementing Multiple Objects
To render multiple objects, we need to make a few adjustments to our code. Let’s modify the TraceRay function inside our Renderer class. First, we need to loop through all the spheres in the scene. For each sphere, we perform the intersection test and update the closest hit position, normal, and color accordingly.
Once we have evaluated all the spheres, we check if we hit anything at all. If not, we return the background color. If we did hit a sphere, we return the color of the closest sphere and perform the lighting calculations.
RayColor Renderer::TraceRay(const Ray& ray, const Scene& scene, const Camera& camera)
{
Sphere* closestSphere = nullptr;
float closestT = FLT_MAX; // Initialize with the maximum possible value
// Loop through each sphere in the scene
for (auto& sphere : scene.spheres)
{
float discriminant;
if (sphere.Intersect(ray, discriminant))
{
// Check if this sphere is the closest so far
if (discriminant > 0 && discriminant < closestT)
{
closestT = discriminant;
closestSphere = &sphere;
}
}
}
// Check if we hit any sphere
if (!closestSphere)
{
return glm::vec4(0.0f, 0.0f, 0.0f, 1.0f); // Return background color
}
// Calculate the hit position, normal, light direction, and color for the closest sphere
glm::vec3 hitPosition = ray.origin + ray.direction * closestT;
glm::vec3 normal = glm::normalize(hitPosition - closestSphere->position);
glm::vec3 lightDirection = glm::normalize(camera.lightPosition - hitPosition);
glm::vec3 color = closestSphere->albedo;
// Perform lighting calculations...
return glm::vec4(color, 1.0f); // Return the color of the closest sphere
}
Rendering Multiple Spheres
With the necessary modifications in place, we can now render scenes with multiple spheres. In the main file, we can create a Scene object and push the desired spheres into it. We can then pass this Scene object to our Renderer along with the camera.
Scene scene;
scene.spheres.push_back(Sphere(glm::vec3(0.0f, 0.0f, 0.0f), 0.5f, glm::vec3(1.0f, 0.0f, 1.0f))); // Pink sphere
scene.spheres.push_back(Sphere(glm::vec3(-5.0f, 0.0f, -5.0f), 1.5f, glm::vec3(0.2f, 0.3f, 1.0f))); // Blue sphere
Renderer renderer;
renderer.Render(scene, camera);
Conclusion
In this episode, we explored the concept of rendering multiple objects in the Ray Tracing series. We discussed how to structure scenes and implemented the necessary code to render scenes with multiple spheres. Feel free to experiment with different scenes and optimize the code further.
Stay tuned for the next episode, where we will restructure our code to prepare for future GPU-based rendering techniques. Don’t forget to check out the code on GitHub and support the series by visiting Techal.
Thank you for joining us, and see you next time!