Data analysis is a critical aspect of any business or research endeavor. It provides valuable insights and helps in making informed decisions. In the realm of data analysis, Python Pandas has emerged as a powerful tool for data manipulation, analysis, and cleaning. In this article, we will explore the various features and functionalities of Python Pandas that make it an integral part of data analysis and data science.
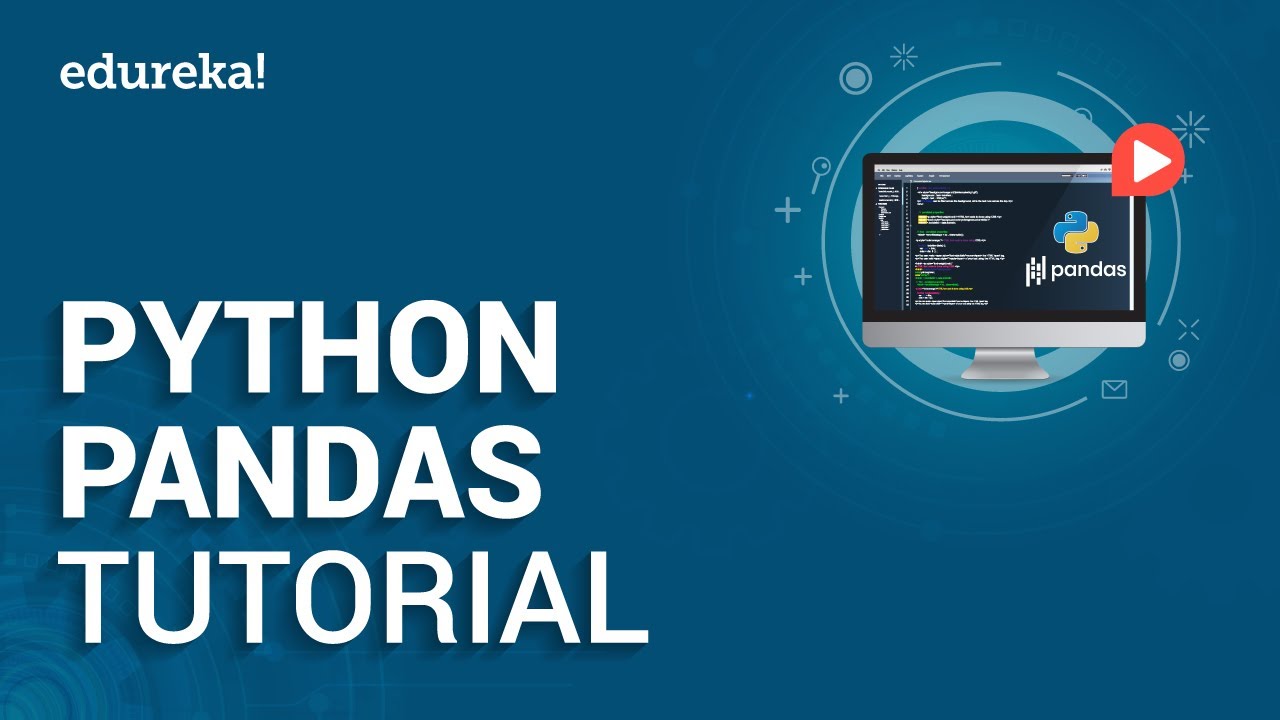
Contents
Introduction
Python Pandas is a Python library that is widely used for data manipulation, analysis, and cleaning. It provides an intuitive and flexible interface for working with structured data. Pandas is well-suited for various types of data, including tabular data with heterogeneous columns, ordered and unordered time series data, arbitrary matrix data, unlabeled data, and other forms of observational or statistical datasets.
Dataframes and Series
At the core of Pandas are two key data structures: Dataframes and Series. A Dataframe is a two-dimensional, mutable, and potentially heterogeneous data structure that represents tabular data. It consists of labeled axes (rows and columns) and supports various operations, such as arithmetic operations aligned on both rows and column labels. A Series, on the other hand, is a one-dimensional labeled array capable of holding data of any type. It can be thought of as a column in an excel sheet.
Let’s take a look at an example. We will create a Dataframe using the Pandas library:
import pandas as pd
# Create a Dataframe
df = pd.DataFrame({'Name': ['John', 'Alice', 'Bob'],
'Age': [25, 30, 35],
'Salary': [50000, 60000, 70000]})
In this example, we have created a Dataframe with three columns: “Name”, “Age”, and “Salary”. Each column contains data of a specific type, such as strings for the “Name” column and integers for the “Age” and “Salary” columns.
We can also create a Series using the Pandas library:
import pandas as pd
# Create a Series
s = pd.Series([1, 2, 3, 4, 5])
In this example, we have created a Series with five elements, each containing an integer value.
Basic Operations
Pandas provides a wide range of operations that can be applied to Dataframes and Series. Let’s explore some of the basic operations.
Viewing Data
To view the data in a Dataframe, we can use the head()
and tail()
methods. The head()
method displays the first five rows of the Dataframe, while the tail()
method displays the last five rows.
# View the first five rows of the Dataframe
df.head()
# View the last five rows of the Dataframe
df.tail()
Selecting Data
To select a single column from a Dataframe, we can use the column name as an index.
# Select a single column from the Dataframe
df['Name']
To slice the rows of a Dataframe, we can use the slicing notation.
# Slice the rows of the Dataframe
df[1:3]
Applying Functions
Pandas allows us to apply functions to Dataframes and Series. We can use the apply()
method to apply a function to each element of a Series or a column of a Dataframe.
# Apply a function to each element of a Series
s.apply(lambda x: x**2)
# Apply a function to a column of a Dataframe
df['Age'].apply(lambda x: x + 10)
Grouping Data
Pandas provides the groupby()
method to group data based on a specific criterion and apply functions to the groups.
# Group data based on the 'Age' column and calculate the mean salary
df.groupby('Age')['Salary'].mean()
Conclusion
In this article, we have explored the various features and functionalities of Python Pandas for data manipulation and analysis. We have learned about Dataframes and Series, basic operations such as viewing data, selecting data, applying functions, and grouping data. Pandas provides a powerful and intuitive interface for working with structured data, making it an essential tool for data analysts and data scientists.
To learn more about Pandas and its advanced features, you can refer to the official Pandas documentation and the Python Pandas tutorial on Techal.
Happy data analysis!