NumPy is a powerful module in Python that is widely used for scientific computing. It offers a wide range of functionalities and tools for efficient data processing, manipulation, and analysis. In this tutorial, we will explore the various operations and functions provided by NumPy arrays.
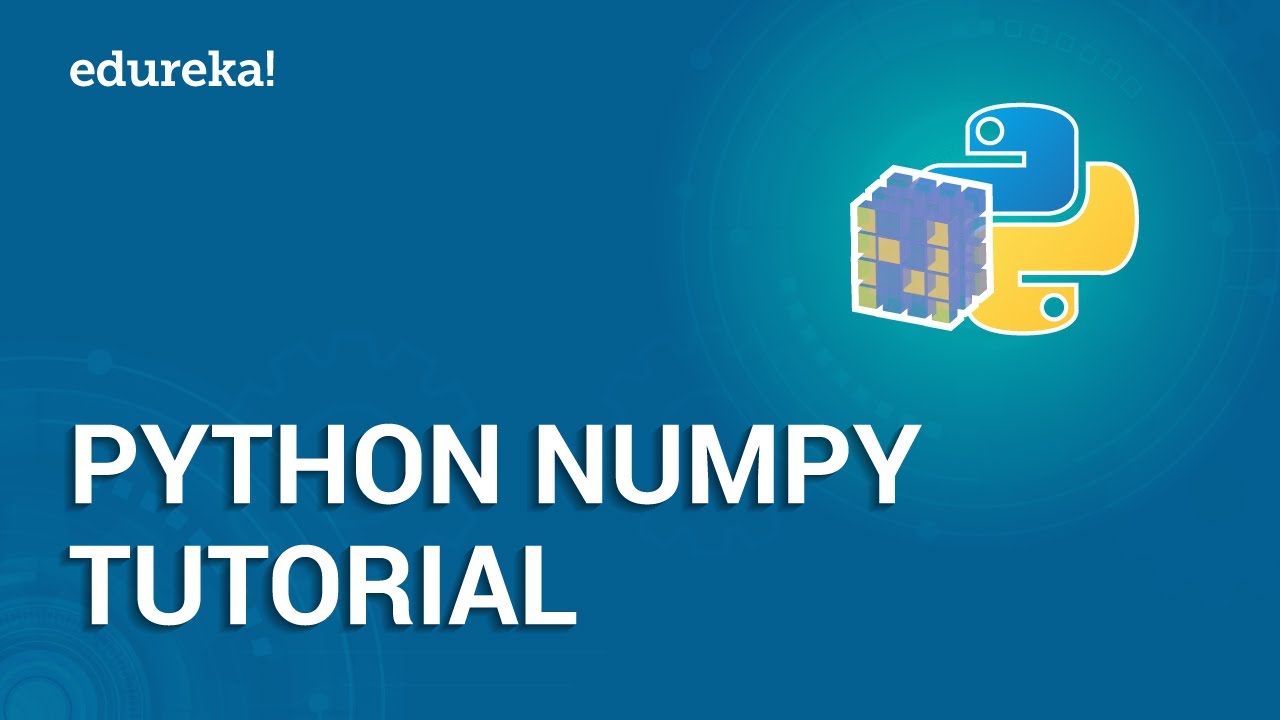
Contents
Introduction
NumPy, short for Numerical Python, is a module in Python that allows for efficient numerical operations and array manipulation. It provides a powerful n-dimensional array object, tools for integrating with other programming languages, and a range of functions for mathematical and logical operations.
In this tutorial, we will cover the following topics:
- Understanding NumPy arrays
- Comparing NumPy arrays with lists
- Performing basic operations on NumPy arrays
- Reshaping and slicing NumPy arrays
- Performing mathematical functions on NumPy arrays
- Working with special functions in NumPy
Understanding NumPy Arrays
A NumPy array is a powerful n-dimensional object that can hold homogeneous data. It offers enhanced mathematical operations and efficient memory management. NumPy arrays can be created using the np.array()
function. Let’s see an example:
import numpy as np
# Create a NumPy array
a = np.array([1, 2, 3, 4, 5])
# Print the array
print(a)
Output:
[1 2 3 4 5]
Here, we have created a one-dimensional NumPy array a
with the elements [1, 2, 3, 4, 5]
. The array can hold any type of numerical data (integers, floating-point numbers, etc.) and can be accessed and manipulated using various operations and functions.
Comparing NumPy Arrays with Lists
NumPy arrays offer several advantages over traditional Python lists:
- Memory efficiency: NumPy arrays occupy less memory compared to lists, as they store data in a contiguous block. This makes them efficient for large datasets.
- Speed: NumPy arrays perform computations faster than lists, as they are optimized for vectorized operations.
- Convenience: NumPy arrays provide a wide range of operations and functions specifically designed for numerical operations, making them convenient to work with.
Let’s compare the memory efficiency and speed of NumPy arrays and lists:
import numpy as np
import time
# Creating a large NumPy array
np_array = np.arange(1000000)
# Creating a large list
list_ = list(range(1000000))
# Comparing memory efficiency
print(np_array.itemsize * np_array.size) # Size of NumPy array in bytes
print(list_.__sizeof__()) # Size of list in bytes
# Comparing computation speed
start = time.time()
np_array += 1
print("Time taken by NumPy array:", (time.time() - start), "seconds")
start = time.time()
list_ = [num + 1 for num in list_]
print("Time taken by list:", (time.time() - start), "seconds")
Output:
8000000 # Memory size of NumPy array in bytes
9000112 # Memory size of list in bytes
Time taken by NumPy array: 0.003 seconds
Time taken by list: 0.532 seconds
As you can see, NumPy arrays occupy less memory and perform computations significantly faster than lists.
Performing Basic Operations on NumPy Arrays
NumPy arrays provide a wide range of mathematical and logical operations that can be performed on the entire array or on specific elements. Let’s explore some of these operations:
import numpy as np
# Create NumPy arrays
a = np.array([1, 2, 3])
b = np.array([4, 5, 6])
# Addition
print(a + b) # [5 7 9]
# Subtraction
print(a - b) # [-3 -3 -3]
# Multiplication
print(a * b) # [4 10 18]
# Division
print(a / b) # [0.25 0.4 0.5]
Output:
[5 7 9]
[-3 -3 -3]
[ 4 10 18]
[0.25 0.4 0.5]
These operations are performed element-wise, meaning that the corresponding elements from both arrays are used for the operation. If the arrays have different shapes, broadcasting rules apply to ensure compatibility.
Reshaping and Slicing NumPy Arrays
NumPy arrays can be reshaped and sliced to extract specific elements or create new arrays with different dimensions. Reshaping involves changing the shape (number of rows and columns) of the array, while slicing involves extracting specific elements or subsets of the array.
import numpy as np
# Create a NumPy array
a = np.array([[1, 2, 3], [4, 5, 6]])
# Reshape the array
b = a.reshape(3, 2)
print(b)
# [[1 2]
# [3 4]
# [5 6]]
# Slicing the array
print(a[:, 1]) # [2 5]
print(a[1, :]) # [4 5 6]
Output:
[[1 2]
[3 4]
[5 6]]
[2 5]
[4 5 6]
Here, we have reshaped the original array a
from a shape of (2, 3)
to (3, 2)
. We have also demonstrated slicing by extracting the second column [2 5]
and the second row [4 5 6]
.
Performing Mathematical Functions on NumPy Arrays
NumPy provides a range of mathematical functions that can be performed on NumPy arrays. Some commonly used functions include calculating the square root, finding the maximum and minimum values, and calculating the sum and mean.
Let’s see how these functions can be applied to NumPy arrays:
import numpy as np
# Create a NumPy array
a = np.array([1, 2, 3, 4, 5])
# Square root
print(np.sqrt(a)) # [1. 1.41421356 1.73205081 2. 2.23606798]
# Maximum value
print(np.max(a)) # 5
# Minimum value
print(np.min(a)) # 1
# Sum and mean
print(np.sum(a)) # 15
print(np.mean(a)) # 3.0
Output:
[1. 1.41421356 1.73205081 2. 2.23606798]
5
1
15
3.0
These functions are applied to each element of the array and return a new array or a single value.
Working with Special Functions in NumPy
NumPy provides several special functions, including trigonometric functions, exponential functions, and logarithmic functions. These functions can be applied to NumPy arrays and provide mathematical solutions to complex problems.
Let’s explore some of these special functions:
import numpy as np
import matplotlib.pyplot as plt
# Create an array of angles
x = np.linspace(0, 2 * np.pi, 100)
# Compute sine and cosine values
y_sin = np.sin(x)
y_cos = np.cos(x)
# Plot the sine and cosine functions
plt.plot(x, y_sin, label="sin")
plt.plot(x, y_cos, label="cos")
plt.legend()
# Display the plot
plt.show()
Output:
A graph showing the sine and cosine functions.
In this example, we have created an array of angles x
using the np.linspace()
function. We have then computed the sine and cosine values of each angle using the np.sin()
and np.cos()
functions. Finally, we have plotted the sine and cosine functions using the plt.plot()
function from the matplotlib
module.
Conclusion
In this tutorial, we have explored the powerful capabilities of NumPy arrays and the various operations that can be performed on them. We have seen how NumPy arrays can be used for efficient data processing, manipulation, and analysis.
By mastering the NumPy module, you can enhance your abilities in scientific computing and gain a deeper understanding of complex mathematical concepts.
To learn more about the functionalities and capabilities of NumPy, and to explore other topics in the realm of technology, visit Techal.
Thank you for reading, and happy coding!