In this article, we will delve deeper into the world of loops and logic in programming. Building upon the foundational knowledge we have gained so far, we will explore more complex structures, step by step.
Before we begin, I encourage you to follow along with pen and paper, tracing through the loops to better understand the logical flow of code. This exercise will help you visualize and comprehend the intricacies of the program.
Let’s jump into a slightly more complicated example. Imagine we have a vector of numbers, ranging from 10 to 50 with an increment of 5. Our goal is to find the squares of each number and store them separately.
To achieve this, we will use a for loop to iterate through the vector, one element at a time. For each iteration, we will square the current element and store the result in a new vector called “a_squared”.
Here’s how the code looks like:
a = [10, 15, 20, 25, 30, 35, 40, 45, 50]
a_squared = [0] * len(a)
for i in range(len(a)):
a_squared[i] = a[i] ** 2
By initializing a new vector, “a_squared,” filled with zeros, we create a placeholder for the squared values. Next, we traverse through each element of “a” using the “for” loop. During each iteration, the value at index “i” is squared and assigned to the corresponding index in “a_squared.”
Executing this code produces an “a_squared” vector containing the squares of the numbers in “a” as expected.
The power of this approach lies in its flexibility. By simply modifying the values in “a,” you can perform different operations while keeping the loop structure intact. For instance, if you change “a” to range from -1 to 1, incrementing by 0.2, the code will adapt accordingly and calculate the squares of the new values.
Now let’s visually represent the relationship between the original vector “a” and the squared values in “a_squared.” Plotting the two vectors on a graph reveals a parabolic curve, demonstrating the expected behavior.
By employing this method, you can easily manipulate and analyze different datasets without rewriting the entire logical structure. Utilizing indices allows for adaptability and efficiency, saving you time and effort.
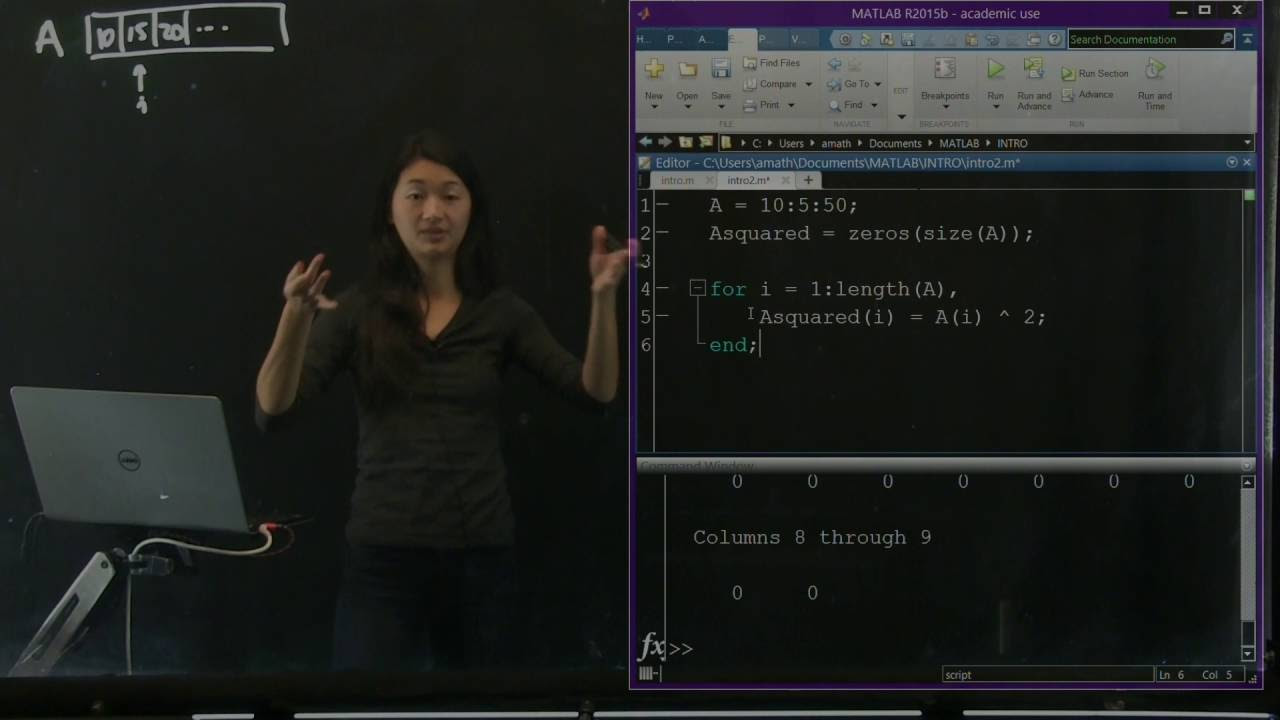
Contents
FAQs
Q: Why do we use a placeholder vector filled with zeros for “a_squared”?
A: Initializing “a_squared” with zeros ensures that it has the same size as the original vector “a.” This one-to-one correspondence guarantees that each element in “a_squared” corresponds to the square of the element in “a.”
Q: Can I perform other operations instead of squaring the values?
A: Absolutely! You can modify the code to perform any desired operation on the elements of “a.” Simply replace the line a_squared[i] = a[i] ** 2
with your desired calculation.
Q: How can I visualize the relationship between the original vector and the calculated values?
A: Plotting the two vectors on a graph provides a visual representation of their relationship. Utilize libraries like Matplotlib in Python to create plots easily.
Conclusion
In this article, we explored advanced concepts related to loops and logic in programming. By leveraging indices and the power of for loops, we can manipulate and analyze data efficiently. By adapting the input vector, we can perform various operations while keeping the logical structure intact.
To learn more about programming and stay updated on the latest technology trends, visit Techal. Keep coding and exploring the endless possibilities of technology!