Welcome to the magical realm of NumPy! In this article, we will explore the power of NumPy, which stands for Numerical Python. NumPy is a library that enables Python to work with large arrays and matrices of numerical data. This is crucial for tasks like computer vision and natural language processing, where data is represented as numerical vectors or matrices.
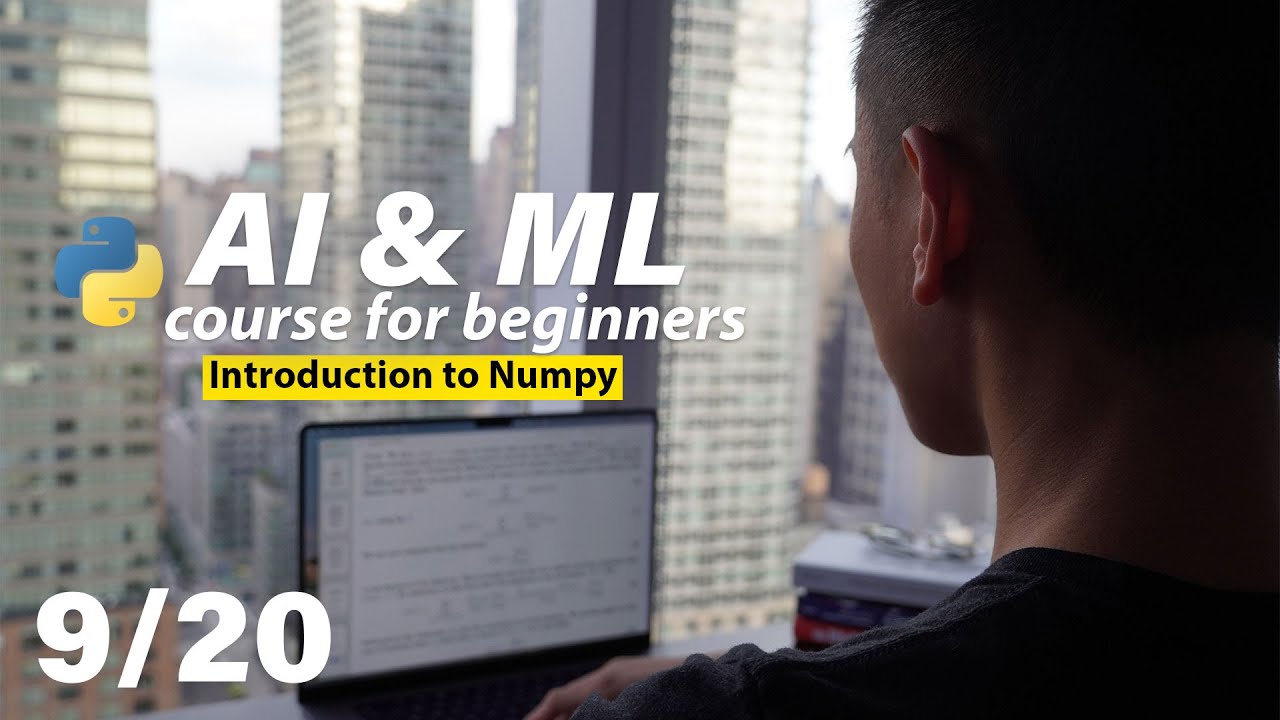
Contents
What is NumPy?
NumPy allows us to store weights, biases, and information within the architecture of neural networks as numbers. It provides the underlying sorcery that makes these concepts possible. With NumPy, we can work with arrays, which are grid-like data structures that store values of the same type. Let’s dive into the code and explore arrays.
Working with Arrays
Arrays are at the heart of NumPy. They can be one-dimensional (1D) or two-dimensional (2D). A 1D array is like a row, while a 2D array is a grid of rows and columns. By nesting arrays, we can create complex structures. Let’s create and print some arrays to understand this better.
import numpy as np
# Creating a 1D array
array_1d = np.array([0, 2, 4, 4, 6])
print(array_1d)
# Creating a 2D array
array_2d = np.array([[0, 2, 4], [4, 6, 8]])
print(array_2d)
Output:
[0 2 4 4 6]
[[0 2 4]
[4 6 8]]
Essential Functions and Operations
NumPy provides several functions and operations that are essential for working with arrays. Here are five of the most important ones:
1. Shape and Reshape
The shape
function allows us to discover the current shape of an array. We can also reshape an array to our liking. The general rule is to use rows, then columns. Let’s see an example:
# Current shape
print(array_2d.shape) # Output: (2, 3)
# Reshaping the array
array_2d_reshaped = array_2d.reshape(3, 2)
print(array_2d_reshaped)
Output:
(2, 3)
[[0 2]
[4 4]
[6 8]]
2. Creating Zero and One Matrices
We can easily create arrays filled with zeros or ones using the zeros
and ones
functions respectively. These are useful for initializing masks and filters:
# Creating a zero matrix
zero_matrix = np.zeros((2, 3))
print(zero_matrix)
# Creating a one matrix
one_matrix = np.ones((2, 3))
print(one_matrix)
Output:
[[0. 0. 0.]
[0. 0. 0.]]
[[1. 1. 1.]
[1. 1. 1.]]
3. Arithmetic Operations
NumPy allows us to perform arithmetic operations on arrays without the need for loops. However, matrices must be compatible for these operations. Let’s see an example:
# Creating arrays
a = np.array([2, 2, 2])
b = np.array([8, 8, 8])
# Adding arrays
c = a + b
print(c)
Output:
[10 10 10]
4. Dot Product
The dot product is a common operation in matrix mathematics. It is crucial for neural network computations. Let’s see an example:
# Dot product
dot_product = np.dot(a, b)
print(dot_product)
Output:
48
5. Slicing Arrays
NumPy allows us to slice arrays to access specific sections. This is useful for examining underlying data. Let’s see an example:
# Creating a matrix
matrix = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
# Slicing the matrix
sliced_matrix = matrix[:2, 1:3]
print(sliced_matrix)
Output:
[[2 3]
[5 6]]
Conclusion
With NumPy, you are equipped to explore the world of AI, computer vision, natural language processing, and more. This powerful library allows you to work with arrays and perform essential operations with ease. Start your AI engineering journey with NumPy and unleash the magic of numerical data!
FAQs
Q: What is NumPy?
A: NumPy is a library that enables Python to work with large arrays and matrices of numerical data.
Q: Why is NumPy important for machine learning?
A: NumPy allows us to represent and manipulate data as arrays, which is crucial for tasks like computer vision and natural language processing.
Q: What are the essential functions and operations in NumPy?
A: Some essential functions and operations in NumPy include shape and reshape, creating zero and one matrices, performing arithmetic operations, computing dot products, and slicing arrays.
Q: How can I reshape an array in NumPy?
A: You can use the reshape
function to change the shape of an array. The general rule is to use rows, then columns.
For more information and resources, visit Techal.