In this article, we will explore the solution to the mean graveyard keeper challenge and discuss an optimized algorithm for adding and subtracting operations. If you have already attempted the challenge, you might have noticed that the naive solution is slow and inefficient. But don’t worry, we will guide you through an optimized approach that reduces the time complexity significantly.
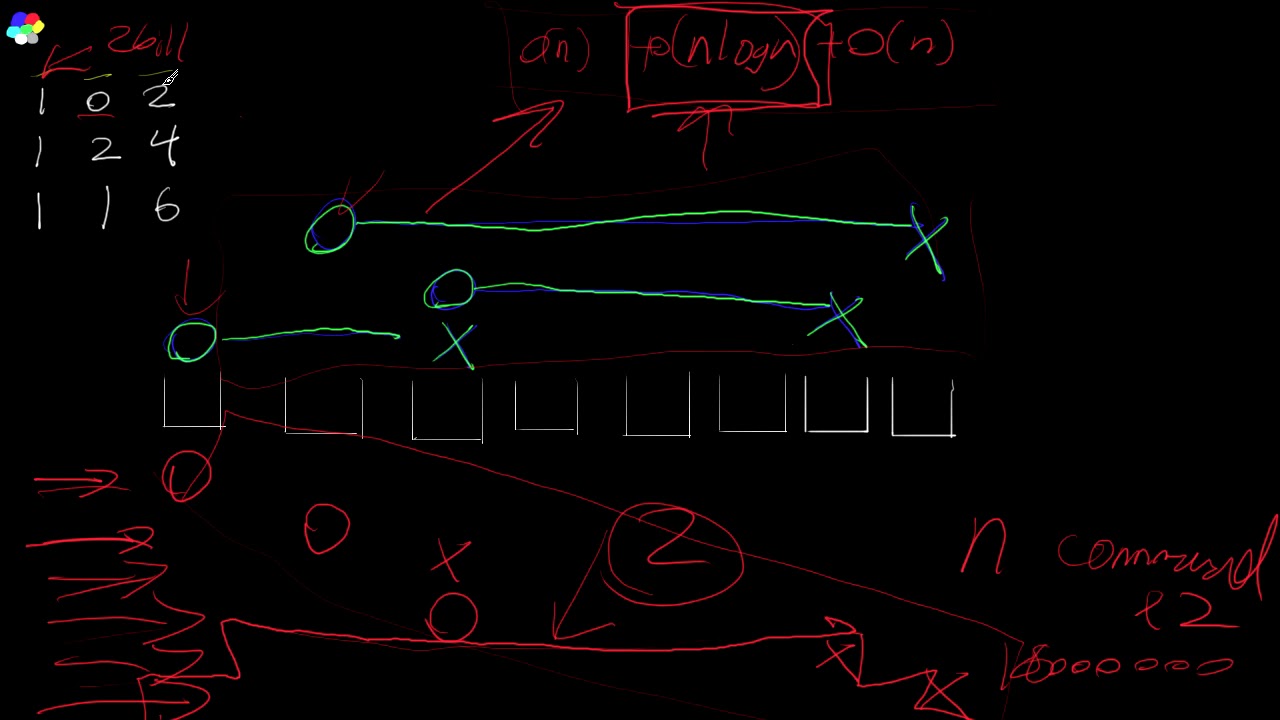
Contents
The Challenge
The challenge involves processing an array of commands, where each command consists of three numbers: the amount to add, the start offset, and the number of buckets. The task is to execute these commands and determine the maximum value among the buckets.
Naive Solution
The naive solution is to iterate over each command and perform the add operation on the corresponding buckets. Finally, we find the maximum value among the buckets. However, this approach is not feasible when the number of buckets is very large, as it would require iterating over billions of elements.
Optimized Solution
To overcome the limitations of the naive approach, we need to develop a more efficient algorithm. Instead of working directly with the buckets, we can work with the commands themselves. Let’s dive into the optimized solution step by step.
Step 1: Create Deltas
First, we create a vector of deltas, which represents the changes in the running total at different positions. Each delta consists of an index and the amount to increase or decrease the running total. We sort these deltas in ascending order based on the index. Sorting is done using an execution policy that utilizes parallelism for faster performance.
Step 2: Process Deltas
Next, we iterate over the sorted deltas and keep track of the running total by adding or subtracting the corresponding amount. We also maintain a maximum value variable to store the highest value encountered during the process. By processing the deltas in the sorted order, we ensure that the additions and subtractions are applied correctly to the running total.
Step 3: Calculate Maximum
Finally, we obtain the maximum value among the buckets by running over the processed deltas. The running total at each position represents the value of the corresponding bucket. We select the maximum value encountered during this process as the result.
Performance Analysis
With the optimized algorithm, the time complexity is reduced to O(NlogN), where N is the number of commands. This is a significant improvement over the O(NF) time complexity of the naive approach, where F represents the number of buckets.
Additionally, we make use of a special case optimization when the number of buckets is very small. In such cases, we utilize multi-threading to process the commands concurrently for improved performance.
Conclusion
By implementing the optimized algorithm, we can efficiently execute the commands and determine the maximum value among the buckets. Remember to use a 64-bit value (long long) for the running total to avoid overflow issues. Additionally, be cautious about the order of deltas when sorting to ensure correct application of additions and subtractions.
If you’d like to delve deeper into the implementation details, you can check out the full code and corresponding explanations provided by the author.
We hope you found this article insightful and learned a new approach to tackle similar challenges. Stay tuned for more exciting coding challenges and solutions by Techal!
FAQs
Q: Can this optimized algorithm be further improved?
A: The algorithm presented here is already optimized for most scenarios. However, if you want to achieve even better performance, you can consider implementing your own sorting algorithm tailored to the specific requirements of the problem.
Q: Why is it important to use a long long data type for the running total?
A: The long long data type provides a larger range of values compared to the standard int data type. Since the running total could potentially exceed the maximum value of an int, using long long ensures accurate calculations without overflow issues.
Q: How does parallel execution improve the performance?
A: Parallel execution takes advantage of multiple cores to process the commands concurrently, resulting in faster execution times. By utilizing the execution policy for parallelism, we can speed up the sorting operation and potentially achieve better overall performance.
Conclusion
In this article, we explored an optimized algorithm for efficiently adding and subtracting values based on a series of commands. By utilizing clever techniques such as sorting deltas and leveraging parallel execution, we can process the commands quickly and accurately determine the maximum value among the buckets.
We hope you found this article informative and enjoyed learning about this efficient algorithm. For more technology-related content and coding challenges, visit Techal and stay tuned for future updates!