Have you ever wondered what exactly “null” means in programming? In managed languages like C#, Java, or even C++, using a null object can result in a null reference or a null pointer exception. This is because these languages are designed to guide developers and provide additional safety measures. But what about C++? Let’s delve into this topic today and explore what the value of null is in C++.
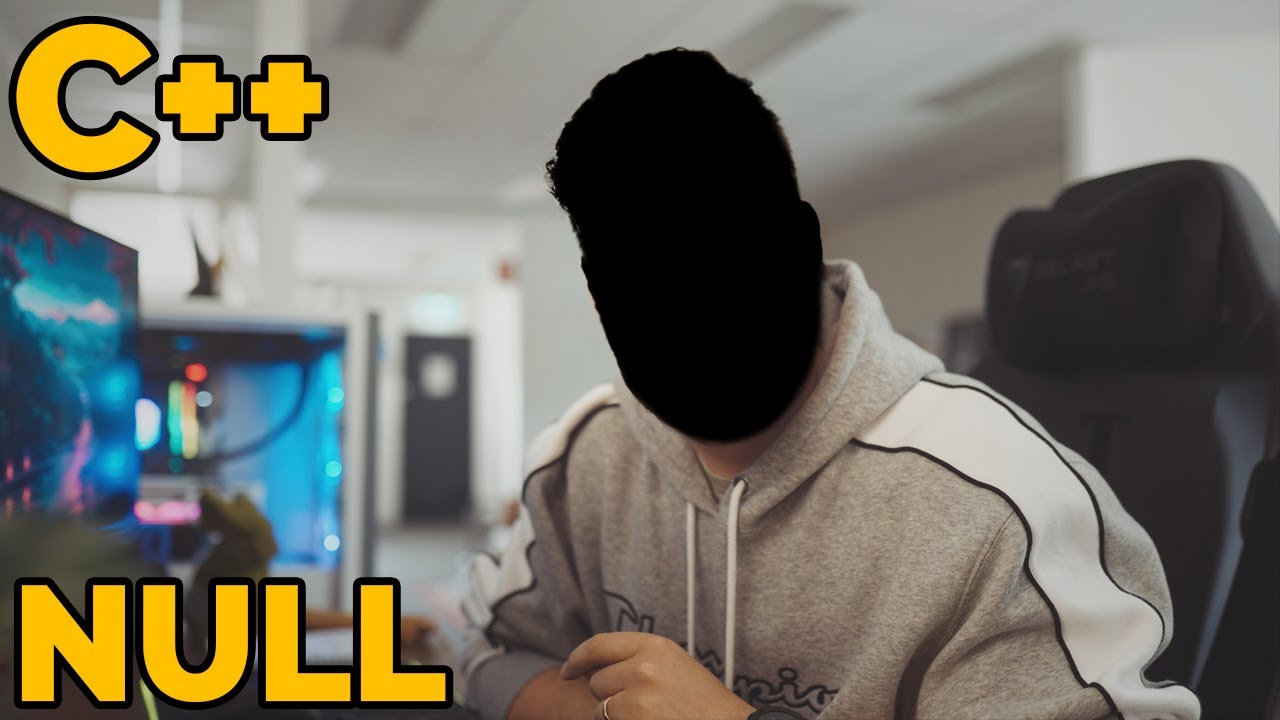
Contents
The Value of NULL in C++
In C++, there is no specific null keyword like in other languages. Instead, C++ uses something called a null pointer. If we take a look at the following line of C++ code:
void* value = nullptr;
We can see that the null pointer is represented by the keyword “nullptr.” When we assign a value to it, such as in the above code, the value stored in the memory address is zero. However, if we examine the actual value in memory, we will see a series of zeros. This is because on a 64-bit architecture, the size of a pointer is 8 bytes. Therefore, null is equal to zero and represents an invalid memory address.
The “Null” Macro in C++
In addition to the null pointer, you may have come across another keyword in C++ called “null.” However, it’s important to note that “null” is not a keyword but a macro. In C, it is commonly used, but it is also acceptable to use it in C++. The macro “null” is defined as zero, just like the null pointer.
Understanding Null in Context
Now, let’s discuss why null can be problematic. In C++, null represents an invalid memory address. When trying to access memory that does not exist or is not valid, you will encounter an access violation. To provide some real-world context, think of it like entering a non-existent address into Google Maps. You won’t be able to reach a location that doesn’t physically exist.
But how does this concept apply to objects in C++? Let’s take a look at an example using a class called “Entity.” In this class, we have two private variables: “parentEntity” and “name.” We also have two member functions: “getName” to retrieve the name and “printType” to print the class type.
If we create an instance of the “Entity” class without initializing a value, it will result in a build error. To avoid this, we should explicitly set the value of the object to the null pointer, as shown below:
Entity* entity = nullptr;
Now, when we try to access the “getName” function, we will encounter a read access violation. This occurs because we are attempting to access a null object, which does not contain valid memory. On the other hand, calling the “printType” function does not crash the program because it does not involve accessing any member variables. Therefore, it is safe to call a function on a null object as long as it does not access any specific instance data.
Understanding Offsets with Null Pointers
To gain a better understanding, let’s discuss the “offsetof” macro in C++. This macro allows us to determine the offset of a member variable within a class. For example, by using the “offsetof” macro, we can find that “MName” in the “Entity” class has an offset of 8 bytes. This means that the first 8 bytes in memory are occupied by the “parentEntity” variable, and the subsequent bytes contain the “MName” variable.
Conclusion
In conclusion, null in programming represents an invalid memory address or the absence of an object. It can lead to crashes or access violations when trying to access specific member variables or functions on a null object in C++. It is essential to understand and handle null values appropriately to avoid runtime errors in your code.
For more exciting tech-related content, visit Techal.
FAQs
Q: What does null represent in programming?
A: Null represents an invalid memory address or the absence of an object in programming. It can cause crashes or access violations when trying to access specific variables or functions on a null object.
Q: How is null different in C++ compared to managed languages like C# or Java?
A: In managed languages, null is explicitly handled to prevent crashes or null reference exceptions. In C++, null is represented by the null pointer and can cause crashes or access violations when accessing specific member variables or functions on a null object.
Q: Why is null used in programming?
A: Null is often used in programming to represent an absence of a value or to initialize variables before assigning them a valid value. It can also be used to check if an object is valid or uninitialized.