Welcome to Techal! In this article, we delve into the world of classes in C programming. If you’re looking to elevate your coding skills, understanding classes is crucial. Let’s explore the power of object-oriented programming and how it can enhance your code organization and functionality.
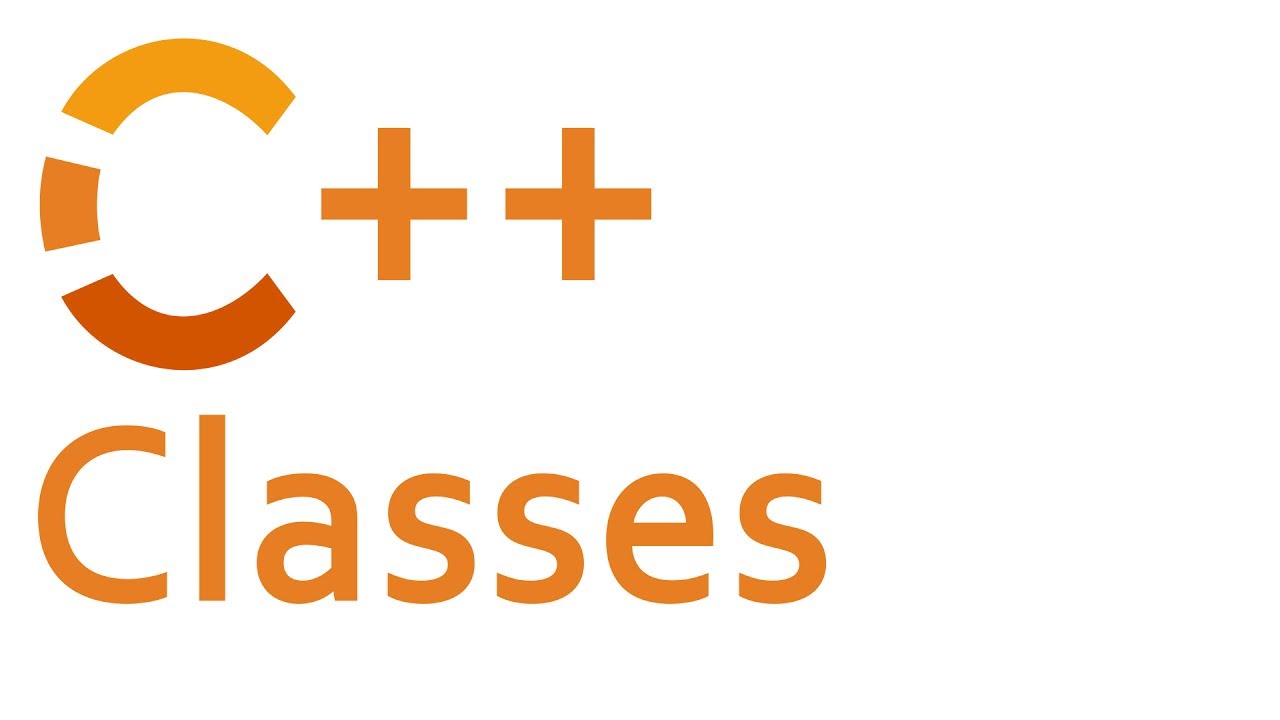
Contents
What are Classes?
Classes are an essential part of object-oriented programming, allowing programmers to group data and functionality together. Think of them as a way to represent real-world objects in your code. For example, consider a player in a game. To fully represent a player, we need data such as position and speed, as well as functionality like movement.
Without using classes, we would have to create individual variables for each piece of data. This approach quickly becomes messy and difficult to maintain. By implementing classes, we can encapsulate all the player-related data and functions into a single unit, making our code cleaner and more organized.
To demonstrate, let’s create a class called Player
. Inside the class, we define the variables we need, such as X
and Y
for position and speed
for movement. By grouping them together, we eliminate the need for numerous individual variables cluttering our code.
class Player {
public:
int X;
int Y;
int speed;
};
Creating Objects with Classes
In order to use the Player
class, we create objects, also known as instances of the class. Each object is a separate entity with its own set of data. To create a Player
object, we write the type Player
followed by a name of our choice.
Player player;
Accessing Class Variables and Methods
We can access the variables and methods of a class using the dot notation. For example, to set the player’s position, we would write player.X = 5
. By default, class variables have private visibility, meaning they can only be accessed within the class itself. To make them accessible outside the class, we need to declare them as public.
class Player {
public:
int X;
int Y;
int speed;
};
int main() {
Player player;
player.X = 5;
player.Y = 3;
player.speed = 2;
// ...
}
Organizing Code with Class Methods
In addition to storing data, classes can also contain functionality in the form of methods. Methods are functions defined inside a class and are used to manipulate the class’s data. For example, let’s create a method called move
that updates the player’s position based on given coordinates.
class Player {
public:
int X;
int Y;
int speed;
void move(int xAmount, int yAmount) {
X += xAmount;
Y += yAmount;
}
};
Now, we can simply call player.move(1, -1)
to move the player one unit to the right and one unit up.
int main() {
Player player;
player.X = 5;
player.Y = 3;
player.speed = 2;
player.move(1, -1);
// ...
}
Simplifying Code with Classes
By utilizing classes, we make our code more organized and easier to maintain. Instead of scattering variables and functions throughout our code, we encapsulate them in a class, providing a cohesive structure for our codebase. This not only enhances readability but also improves code efficiency and encourages modular development.
FAQs
Q: Can I use classes in C programming without a specific object-oriented programming language?
A: Yes, you can. While languages like C++ and Java are primarily object-oriented, C also supports classes. However, it’s important to note that classes in C are not as robust as those in dedicated object-oriented languages.
Q: Are classes mandatory for writing code in C?
A: No, classes are not mandatory. C is a perfectly usable language without classes. However, classes provide a cleaner and more organized way of programming, making code maintenance and development more manageable.
Q: Can I achieve the same functionality without using classes?
A: Yes, you can. Anything you can do with classes, you can also do without them. Classes are a tool to make your programming life easier by providing syntactic sugar and code organization.
Conclusion
Classes are a powerful tool in C programming that empowers developers to structure their code, enhance functionality, and improve code maintenance. By encapsulating data and functionality within a class, we create clean and efficient code. We hope this article has provided you with a solid foundation for utilizing classes in your own projects.
If you’re interested in exploring more about C programming, feel free to visit our website Techal for additional guides, tips, and insights. Stay tuned for future articles where we dive deeper into the world of classes and learn advanced concepts to level up your coding skills. Happy coding!