Welcome back to the series where I remake my first Java game in C++. Today, we will focus on rendering tiles and Sprites. In the previous episode, we successfully loaded levels. Now, let’s dive in and take a closer look at rendering.
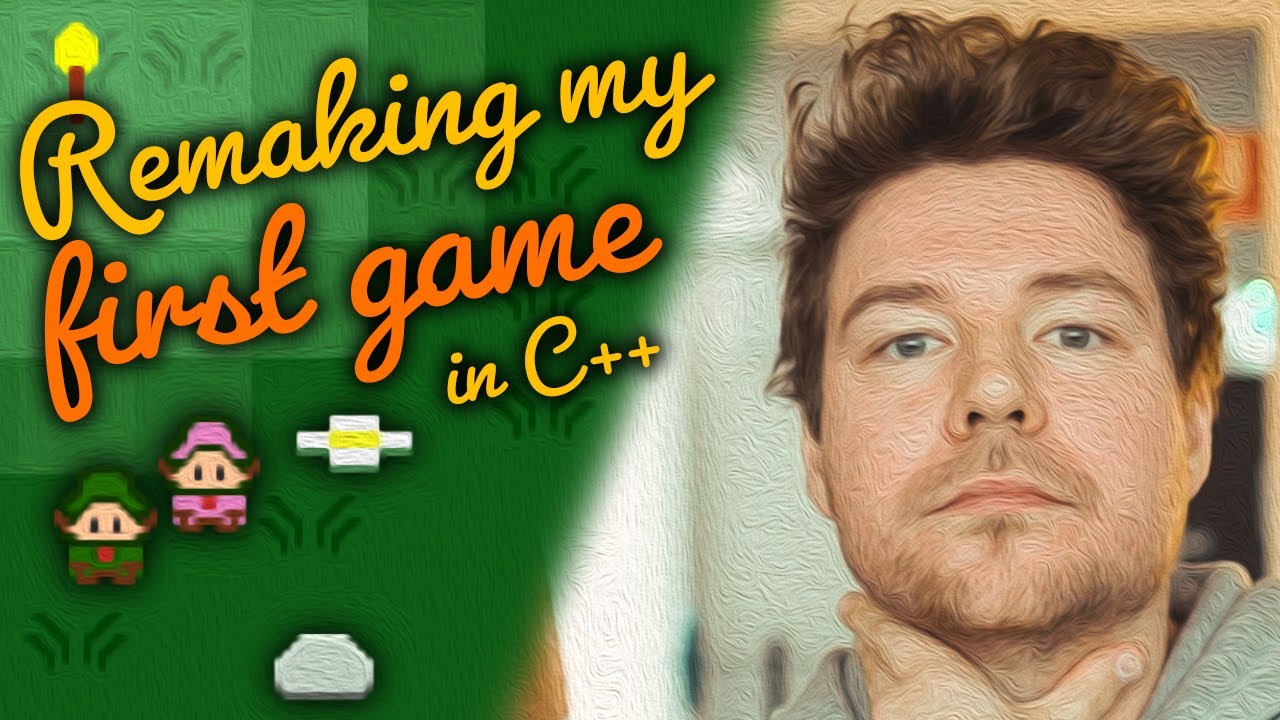
Contents
Understanding the Rendering Process
In our current C++ code, we are loading and displaying a level by copying the pixels directly onto the screen. However, we need to go a step further and read the color of each pixel to determine the corresponding tile in the world. Additionally, we only want to render the tiles that are within the camera viewport.
Improving the Rendering Function
Instead of using a switch statement and hardcoding the tile IDs, let’s create a more flexible and intuitive function. We can create a getTileSprite
function that takes the X and Y coordinates as parameters and returns the appropriate Sprite for that tile.
Sprite getTileSprite(int x, int y) {
// Retrieve the tile ID at the given coordinates
int tileID = getTile(x, y);
// Assign the appropriate Sprite based on the tile ID
switch(tileID) {
case 0x00:
return Sprites::grass;
case 0x01:
return Sprites::water;
// Add more cases for other tile types...
}
// If the tile ID is invalid, return the water Sprite as a fallback
return Sprites::water;
}
By using this function, we can easily determine the Sprite for a tile based on its coordinates. For example, if the tile ID at (x, y)
equals 0x00
, we return the grass Sprite. If it equals 0x01
, we return the water Sprite. This makes the code more readable and easier to maintain.
Rendering Multiple Tiles
Now that we have an improved rendering function, let’s loop through the entire level to render all the tiles. We can iterate over each tile and call our drawSprite
function to render the appropriate Sprite at the correct position.
for (int y = 0; y < levelHeight; y++) {
for (int x = 0; x < levelWidth; x++) {
// Get the Sprite for the current tile
Sprite tileSprite = getTileSprite(x, y);
// Calculate the screen position to render the tile
int screenX = x * tileSize;
int screenY = y * tileSize;
// Render the tile Sprite at the screen position
drawSprite(screenX, screenY, tileSprite);
}
}
In this loop, we iterate over the coordinates of each tile in the level. We retrieve the corresponding Sprite using our getTileSprite
function and calculate the screen position using the tile size. Finally, we call drawSprite
to render the tile Sprite at the correct position on the screen.
Conclusion
In this episode, we focused on rendering tiles and Sprites in our game. We improved the rendering function by using a more intuitive getTileSprite
function, allowing us to easily retrieve the Sprite based on the tile’s coordinates. We also looped through the entire level to render multiple tiles using the updated rendering function.
In the next episode, we will explore implementing a camera system to enable movement within the game world. Stay tuned for more updates and improvements to our remade game in C++!
Check out more articles and tutorials on the Techal website!