Welcome back, everyone! Today, we are going to dive into the fascinating world of 2D arrays in C++. Specifically, we will explore how to efficiently implement 2D arrays in game programming and overcome challenges such as circular dependency. Let’s get started!
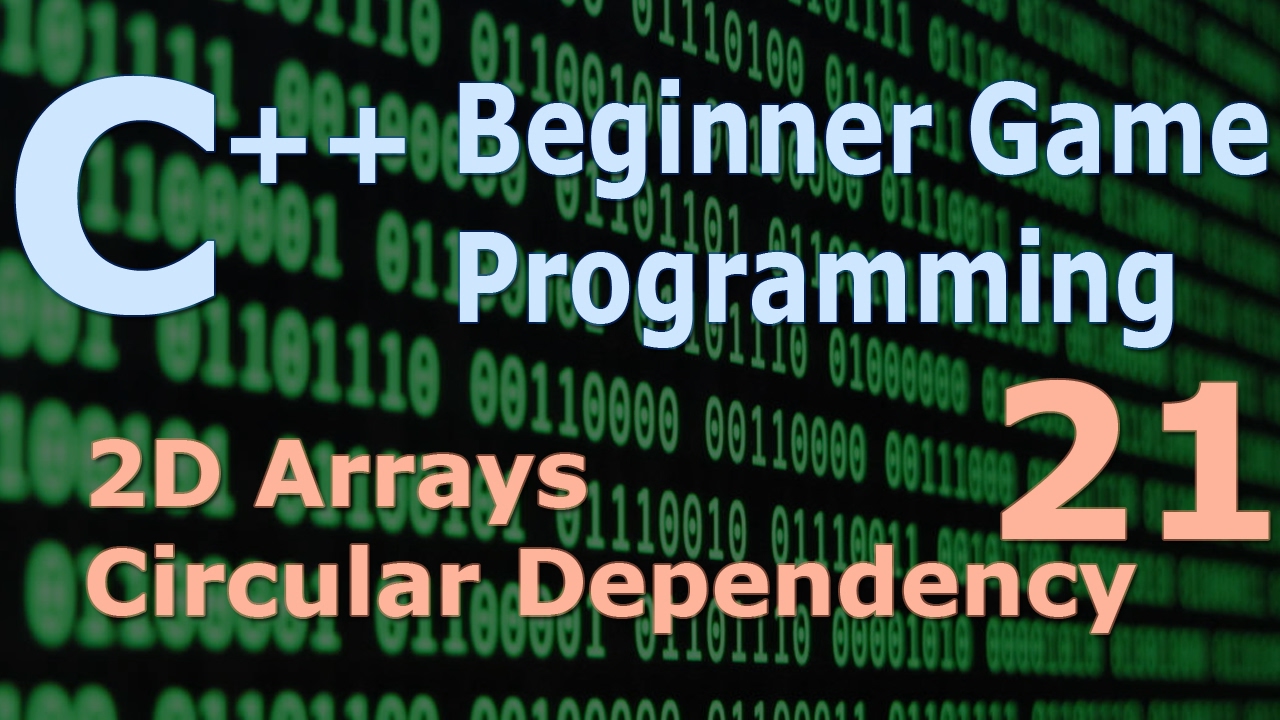
Contents
Motivation: Adding 2D Arrays to the Snake Game
To start, let’s discuss the motivation behind incorporating 2D arrays into our game. In a previous tutorial, we introduced the concept of the snake game and challenged you to add random obstacles to the game field. Up until now, most of you have likely stored these obstacles in a large array and checked for collisions during the snake’s movement. However, this approach can become inefficient when dealing with a large number of obstacles.
For example, imagine a game board with dimensions of 100×100 and one-third of the board filled with obstacles. Each time you move the snake, you would have to check all 3,000 obstacles to see if there is a collision. This process can quickly become wasteful, even though computers can handle it effortlessly.
So, what if we could optimize this process and make it more efficient? That’s where 2D arrays come in!
Efficiently Handling Obstacles with 2D Arrays
Instead of storing obstacles in a separate array, we can implement a 2D array that mirrors the dimensions of the game board. In this 2D array, each element corresponds to a location on the game board and can store information about whether an obstacle is present.
To achieve this, we need to map the 2D coordinates (X, Y) to a single index in the 1D array. This mapping allows us to access the necessary elements efficiently. Let’s dive into the details!
Mapping 2D Coordinates to 1D Array
Let’s assume we have a 4×3 game board. We can visualize this 2D array as follows:
0 1 2 3
4 5 6 7
8 9 10 11
Now, if we number the elements in the 2D array from left to right, top to bottom, we get:
0 1 2 3 4 5 6 7 8 9 10 11
Notice how the numbers in the 2D array line up with the elements in the 1D array.
To determine the index in the 1D array based on the 2D coordinates (X, Y), we can use the following formula:
index = Y * width + X
Here, width
refers to the width of the 2D array.
For example, if we want to access the element at coordinates (3, 2), the corresponding index in the 1D array would be:
index = 2 * 4 + 3 = 11
This mapping allows us to efficiently access elements in the 1D array, reducing the number of checks required when moving the snake.
Implementing 2D Arrays in C++
To implement this mapping in C++, we first need to create a 1D array and set its size to the width times the height of the game board. We can then initialize all the elements in the array to false
, indicating no obstacles present.
Next, we can create a function that checks whether a given location contains an obstacle. This function will convert the 2D coordinates (X, Y) to the corresponding index in the 1D array and return the value at that index.
Additionally, we should include a function to spawn random obstacles on the game board. We can generate random locations and check if those locations are free from both the snake and existing obstacles. Once we find a suitable location, we mark it as containing an obstacle.
Lastly, we need a function to draw the obstacles on the game board. We can loop through the 2D coordinates and check if each location contains an obstacle. If it does, we draw a gray rectangle to represent the obstacle.
Conclusion
In this tutorial, we explored the implementation of 2D arrays in game programming, specifically in the context of the snake game. By efficiently mapping these arrays onto 1D arrays and utilizing the power of row-major mapping, we can enhance the performance of our game and handle obstacles more efficiently.
We also discussed the concept of circular dependency and how to overcome it using forward declaration. This technique allows classes to refer to each other without causing compilation errors.
By applying the knowledge gained in this tutorial, you can optimize your game development process and create more engaging experiences for your players. Remember, the possibilities in the world of C++ game programming are endless!
If you have any questions or comments, feel free to join our Discord community or leave a comment on the YouTube video. We would love to hear from you.
Thank you for joining us on this exciting journey into the world of C++ game programming. Stay tuned for more tutorials and explore the Techal brand at Techal.
FAQs
Q: Can I use other methods to implement 2D arrays?
A: Yes, there are alternative methods to implement 2D arrays, but mapping the 2D array to a 1D array using row-major mapping offers the greatest flexibility and efficiency.
Q: How do I handle circular dependencies in C++?
A: To handle circular dependencies, use forward declaration. Include the necessary headers in the appropriate CPP files and rely on forward declaration for function declarations.
References
- Techal: Official website of the Techal brand.
- C++ Game Programming Tutorial: A comprehensive video tutorial series on C++ game programming by Techal.