Word embeddings play a crucial role in Natural Language Processing (NLP), enabling machines to understand the meaning behind words and derive relationships between them. In this article, we will explore word embeddings in Gensim, a popular Python library primarily used for topic modeling.
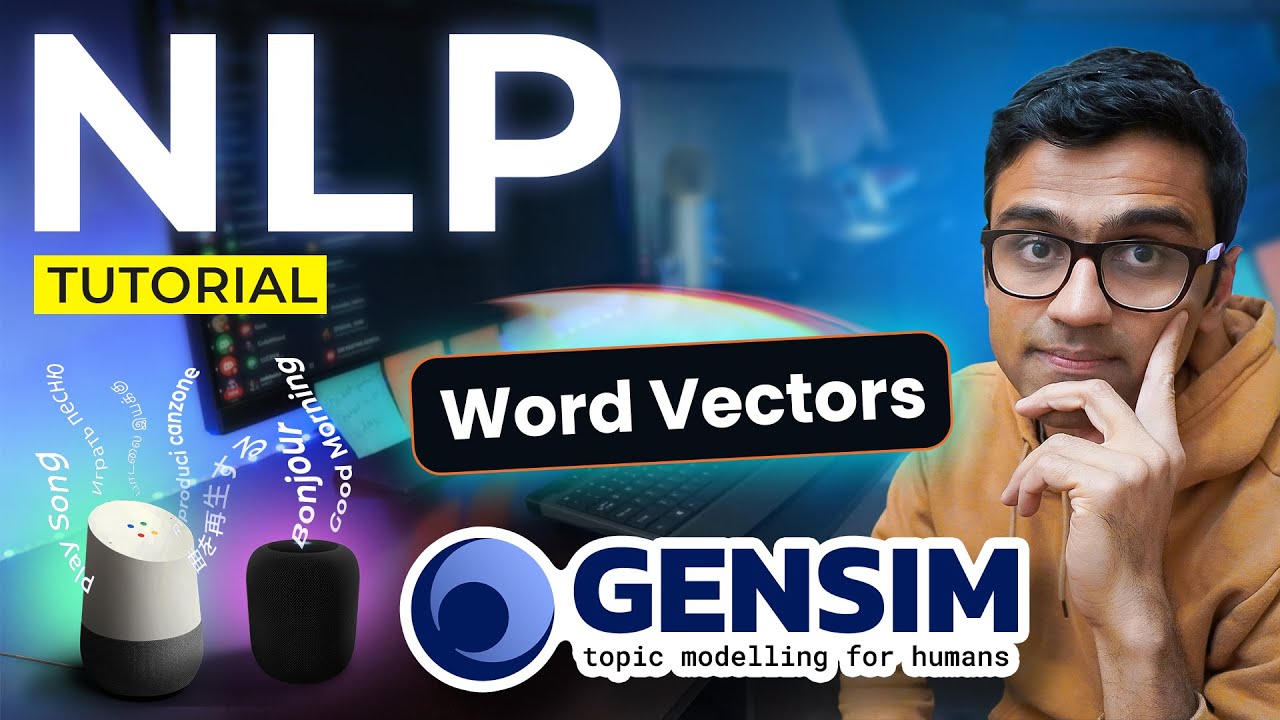
Contents
An Introduction to Gensim
Gensim is a powerful NLP library similar to Spacy. While we will cover topic modeling in a future article, today we will focus on Gensim’s word vectors. One advantage of Gensim’s API is its convenience when working with word vectors. Before we delve into the details, it’s important to note that Gensim’s documentation can be found on their website.
Loading Word Vectors in Gensim
To get started, install Gensim by running the command pip install Gensim
. Once installed, you can import the necessary word vectors from the Gensim library using the following code snippet:
import Gensim
from Gensim import downloader
# Load the appropriate word vectors
word_vectors = downloader.api.load("word2vec-google-news-300")
Gensim offers various word embeddings, including models trained on Google News, GloVe, and Twitter datasets. For example, the “word2vec-google-news-300” model weighs around 1.6 gigabytes and is trained on 100 billion words. It contains approximately 3 million word vectors. If you require a smaller model, there are options available as well, such as the “glove-twitter-25” model, weighing only 27 megabytes and trained on 2 billion tweets.
Exploring Word Similarity and Relationships
Once you have loaded the word vectors, you can explore the similarities and relationships between words. Gensim provides convenient functions to accomplish this. For instance, the similarity()
function allows you to measure the similarity between two words. Consider the code snippet below:
similarity_score = word_vectors.similarity("great", "good")
print(similarity_score)
The output of the above code will be a similarity score between the words “great” and “good.” A score of 1 indicates identical meaning, while lower values indicate less similarity.
You can also utilize the most_similar()
function to find words that are most similar to a given word. The example below demonstrates finding words similar to “good” using Gensim:
similar_words = word_vectors.most_similar(positive=["good"])
print(similar_words)
Similarly, you can perform operations such as King - man + woman = Queen
, as shown in the following code:
result = word_vectors.most_similar(positive=["king", "woman"], negative=["man"])
print(result)
This operation results in the word vector closest to the calculated result, which happens to be “queen” in this case.
Gensim also offers an interesting function called doesnt_match()
, which helps determine which word in a set does not fit with the others. For example:
outlier = word_vectors.doesnt_match(["apple", "banana", "carrot", "door"])
print(outlier)
The output will identify the word that doesn’t match the others, in this case, “door.”
FAQs
Q: Which datasets are available for word vectors in Gensim?
Gensim provides various pre-trained word vectors, including models trained on Google News, GloVe, and Twitter datasets. These datasets differ in size and the number of vectors they contain.
Q: How can word vectors be used in practical applications?
Word vectors are widely used in NLP tasks such as sentiment analysis, text classification, and information retrieval. By representing words in vector form, machines can understand semantic relationships between words and derive meaning from text.
Q: Can Gensim be used for topic modeling?
Yes, Gensim is an excellent choice for topic modeling. Although we haven’t covered it in this article, we will explore topic modeling using Gensim in future tutorials.
Conclusion
Word vectors are a powerful tool in NLP, enabling machines to understand the relationships and meanings behind words. In this article, we introduced Gensim, a popular Python library used for topic modeling. We explored how to load word vectors in Gensim and perform various operations such as measuring similarity, finding similar words, and determining outliers. By leveraging the capabilities of Gensim, you can unlock the potential of word vectors in your NLP projects.
If you enjoyed this article, feel free to share it with your friends and colleagues. Don’t forget to follow Techal for more informative content on the ever-evolving world of technology.