Welcome back to the Techal Say Plus Plus series! Today, we’ll dive into the fascinating world of the mutable
keyword in C++. You may be wondering, what does this keyword do? Well, let’s uncover its secrets and explore how it can be used in two distinct scenarios.
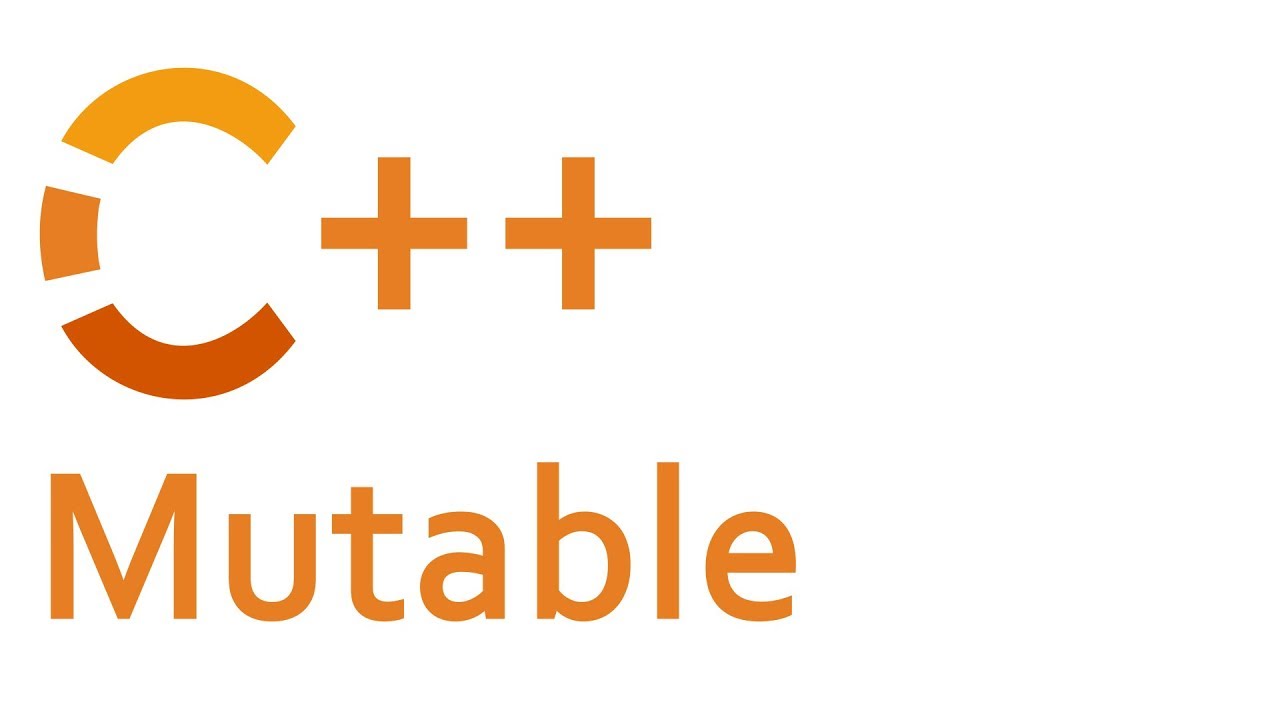
The Power of Mutable with Constants
In C++, the const
keyword is used to declare constants, indicating that the value of a variable cannot be changed. But what if you want to modify a variable within a const
method? This is where mutable
comes in handy.
Consider a class called Entity
with a private member variable name
. To access this variable, we define a const
method called get_name()
. By marking this method as const
, we promise not to modify any class members. However, there are situations where we might want to modify a non-member variable, like a debugging counter, within this method.
Here’s an example:
class Entity {
private:
std::string name;
mutable int debug_count = 0; // Mutable variable for debugging purposes
public:
const std::string& get_name() const {
debug_count++; // Increment the debug count
return name;
}
};
By declaring the debug_count
variable as mutable
, we allow const
methods to modify it, while still maintaining the immutability of other class members. This usage of mutable
with class members is the most common scenario you’ll encounter.
Mutable and Lambdas
In addition to its role with constants, the mutable
keyword can also be used with lambdas. A lambda is a small throwaway function that can be assigned to a variable quickly.
To understand mutable
in the context of lambdas, imagine we have a variable x
with the value “Sarah”. We then define a lambda called my_lambda
that does something with x
. By default, lambdas capture variables by value, meaning they make a copy of the variable.
std::string x = "Sarah";
auto my_lambda = [=]() { // Capture 'x' by value
std::cout << x << std::endl;
};
But what if we want to modify x
within the lambda, even though we’re capturing it by value? This is where mutable
comes to the rescue. By adding the mutable
keyword to the lambda, we can modify variables captured by value without altering the original variable.
std::string x = "Sarah";
auto my_lambda = [=]() mutable { // Make the lambda mutable
x += " is awesome!";
std::cout << x << std::endl;
};
In this example, x
will still be “Sarah” after calling the lambda because the modification happens within the lambda’s local scope.
FAQs
Q: When should I use the mutable
keyword with constants?
A: The mutable
keyword is primarily used with class members inside const
methods when you need to modify non-member variables or perform debugging operations without violating the immutability of the class.
Q: Are there any other practical uses of the mutable
keyword?
A: While the majority of mutable
use cases involve class members, you can also use it with lambdas to modify variables captured by value without altering the originals.
Q: Should I use the mutable
keyword with lambdas regularly?
A: In practice, using mutable
with lambdas is not very common. It’s mostly used when you need to modify a value captured by value within the lambda’s local scope.
If you have any additional questions about the mutable
keyword or constants in C++, feel free to leave a comment below!
Conclusion
The mutable
keyword in C++ is a powerful tool that allows for the modification of variables within const
methods and lambdas. By selectively marking variables as mutable
, you can maintain the immutability of class members while still making necessary modifications.
To learn more about C++, don’t forget to check out Techal for informative articles, comprehensive guides, and the latest updates in the world of technology. Happy coding!