Welcome back to the Techal C++ series! Today, we’re going to dive into the concept of singletons in C++ – a design pattern rather than a language feature. So, what exactly is a singleton and why should you care? Let’s find out!
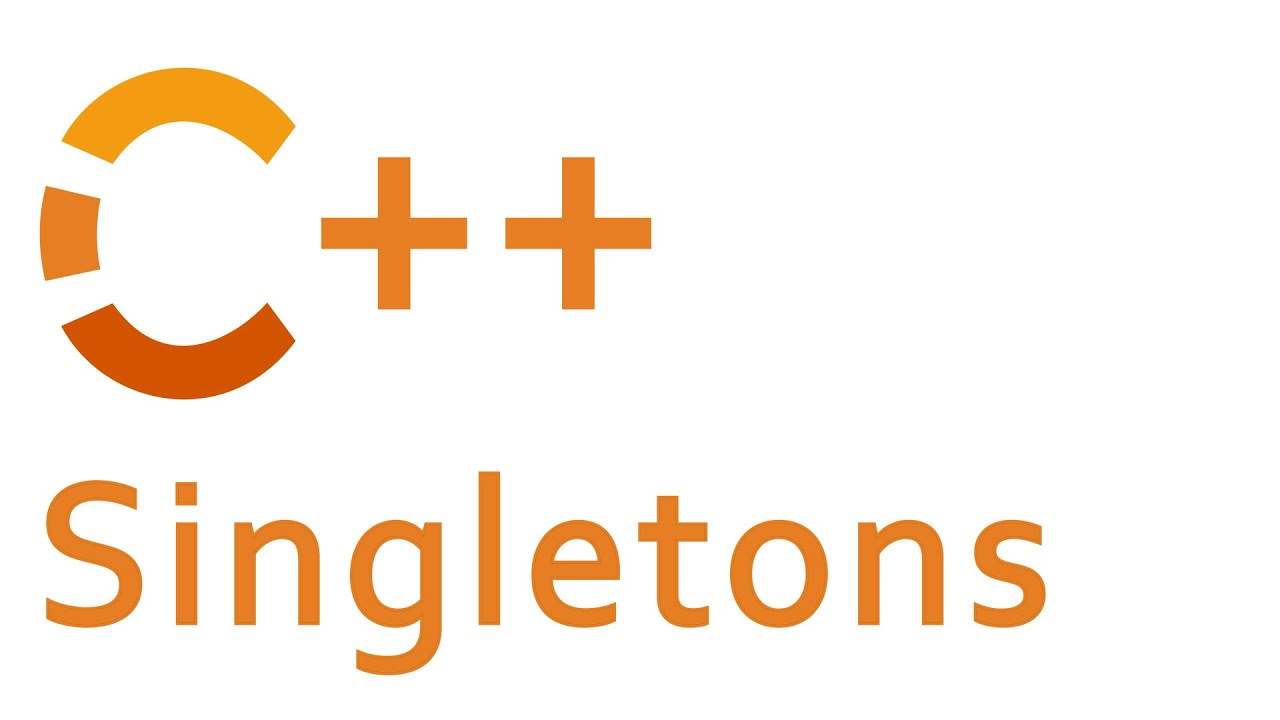
Contents
What is a Singleton?
In simple terms, a singleton is a single instance of a class that you keep around throughout your application. It is meant for situations where you only need one instance of a class or struct. Think of it as a way to organize a set of global variables and static functions into a single, organized package.
Examples of Singletons
Let’s look at a couple of examples to understand how singletons can be useful.
Random Number Generator: Suppose you have a class that generates random numbers. Instead of creating multiple instances of this class, you can use a singleton to have a single instance that you can query for random numbers.
Renderer: Another example is a renderer, which is often a global entity in many applications. Instead of having multiple instances of a renderer, you can use a singleton to have a single instance that handles rendering for your entire application.
Implementing a Singleton in C++
To implement a singleton, here’s a basic approach you can follow:
- Make the constructor private, ensuring that the class cannot be instantiated outside of itself.
- Provide a static function, commonly named
getInstance()
, that returns a reference or pointer to the singleton instance. - Create a static member variable inside the
getInstance()
function to hold the singleton instance. This variable will be initialized on its first use and then reused throughout the application.
Here’s an example code snippet to illustrate the implementation of a singleton:
class Singleton {
private:
Singleton() {} // Make the constructor private
static Singleton& getInstance() {
static Singleton instance; // Create the static instance
return instance;
}
public:
void doSomething() {
// Do something with the singleton instance
}
};
To use the singleton, you can simply call the getInstance()
function and access the methods and members through the returned instance:
Singleton::getInstance().doSomething();
Why Use a Singleton?
Singletons can be a useful tool in your coding toolbox, especially in scenarios where you want to have a single instance of a class that is globally accessible. They help you organize your code and provide an easy way to access shared resources.
Conclusion
Singletons are a design pattern in C++ that allow you to have a single instance of a class throughout your application. They can be useful in situations where you only need one instance of a class or when you want to organize global resources.
Keep in mind that while singletons can be helpful, they should be used judiciously and with caution. So, the next time you find yourself needing a single instance of a class, consider implementing a singleton!
FAQs
Q: Can’t we achieve the same functionality with a namespace instead of a class?
A: Yes, you can technically achieve similar functionality using a namespace. However, using a class allows for better organization and access control, making it a preferred choice for many developers.
Q: When should I use a singleton?
A: Singletons are typically used when you want to have a single instance of a class that can be accessed globally. It’s important to use singletons judiciously and consider alternative design patterns or approaches when appropriate.
Q: Are singletons thread-safe?
A: The implementation of singletons can be made thread-safe by using techniques such as double-checked locking or atomic operations. However, thread safety is beyond the scope of this article and should be carefully considered based on your specific requirements.
Q: Can I extend a singleton class?
A: Yes, you can extend a singleton class like any other class. However, keep in mind that the singleton pattern restricts the number of instances to one, so any subclasses will also be limited to a single instance.
Thanks for joining us for this exploration of singletons in C++. We hope you found this article informative and useful. If you have any questions or thoughts, feel free to leave them in the comments below. Stay tuned for more informative content from Techal!
-Image Credit: Techal