Welcome to episode 120 of Game Programming! After a brief hiatus due to illness, we are back and more determined than ever to make progress on our game. Today, we will focus on polishing the UI buttons, fixing bugs, and improving usability. Let’s dive right into it!
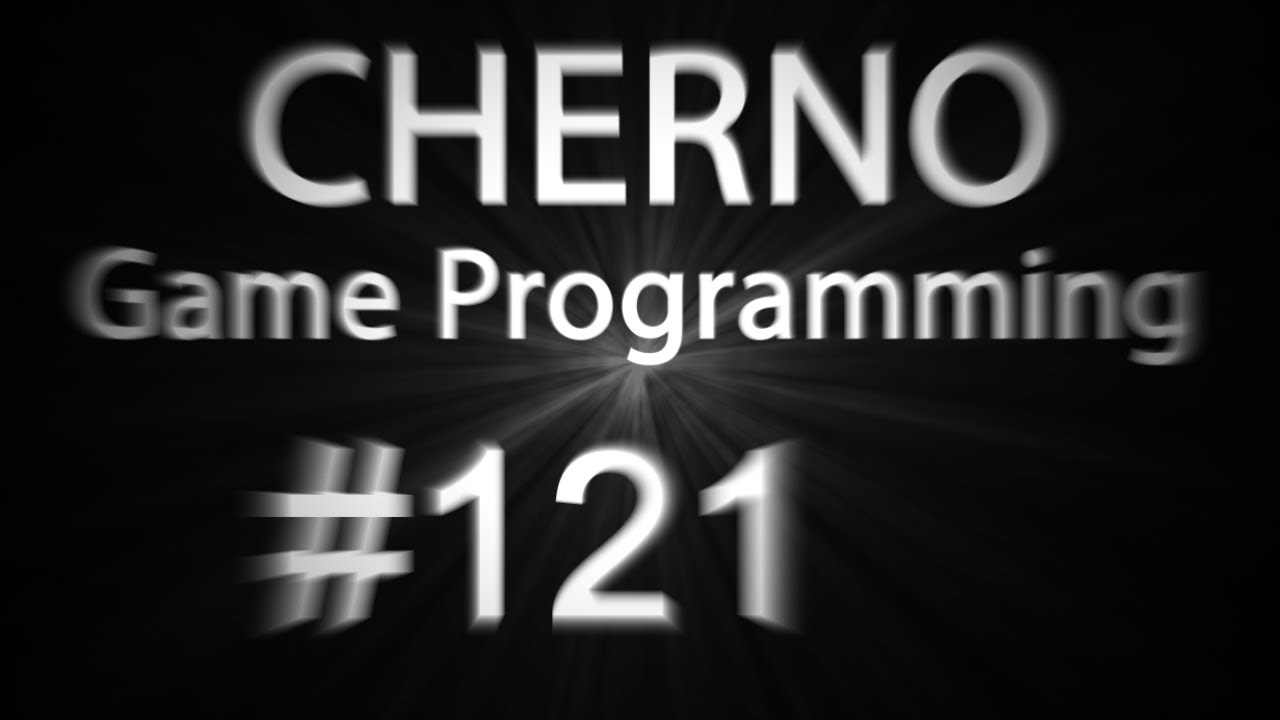
One of the bugs we have encountered is that when the mouse is pressed and dragged outside of the button, it still registers as a click. This behavior is not desired, as the button should only be considered pressed when the mouse is both inside the button and actively being held down.
To fix this, we need to detect whether the mouse was pressed when it was inside the button. By adding a check for the left mouse button being down, we can ensure that the button is only pressed when the mouse is inside it.
Here’s what the code looks like:
if (!pressed && !ignorePressed && leftMouseButton.isDown()) {
// button is pressed
// perform actions
}
With this code in place, the button will only be considered pressed if the left mouse button is down, and if the button was entered while already pressed. This fixes the bug and ensures that the button behaves as expected.
We also wanted to improve the API for our UI buttons. We made it possible to override the default behavior of the pressed button action by setting our own action. However, we noticed that calling the performAction method directly would result in the action being performed twice, once during the press and once during the release.
To solve this issue, we introduced a boolean flag called “ignoreAction”. By setting this flag to true, we can prevent the next action from being performed. This allows us to control when the action should be executed and avoid unnecessary duplication.
Here’s an example of how to use the “ignoreAction” flag:
button.setButtonListener(new UIButtonListener() {
@Override
public void pressed() {
button.ignoreNextPress(); // ignore the next action
// your custom code here
}
});
With this improvement, we have created a more flexible and customizable API for our UI buttons.
In our next episode, we will cover the process of adding images to our UI buttons. This will allow us to enhance the visual appeal of our game and create more engaging user interfaces.
FAQs
-
Can I customize the behavior of the button press action?
Yes! You can override the default behavior by setting your own action using thesetButtonListener
method. This allows you to perform custom actions when the button is pressed. -
Is it possible to prevent duplicate actions when calling
performAction
directly?
Yes! By using theignoreNextPress
method, you can prevent the next action from being performed. This gives you control over when the action should be executed.
Conclusion
In this episode, we focused on polishing our UI buttons in game programming. We fixed bugs, improved the API, and discussed upcoming topics like adding images to buttons. We hope this guide has provided you with valuable insights and empowered you to create better user interfaces for your games. Stay tuned for the next episode!
If you enjoyed this article, please support us on Techal and consider joining our Patreon for exclusive content and personalized support. Your contributions help us continue creating informative and engaging content. Thank you for your support!