As technology enthusiasts and engineers, we often find ourselves wanting to analyze and visualize large amounts of data. In the previous sections, we discussed various techniques for plotting data, including multiple plots on top of each other. While this approach can be useful for comparing data and identifying trends, it can also become confusing when dealing with a massive number of plots.
In this guide, we will explore a simple and efficient way to plot a large array of plots simultaneously. This technique allows us to save time and avoid repetitive coding. We will also learn how to customize our plots to make them more informative and visually appealing.
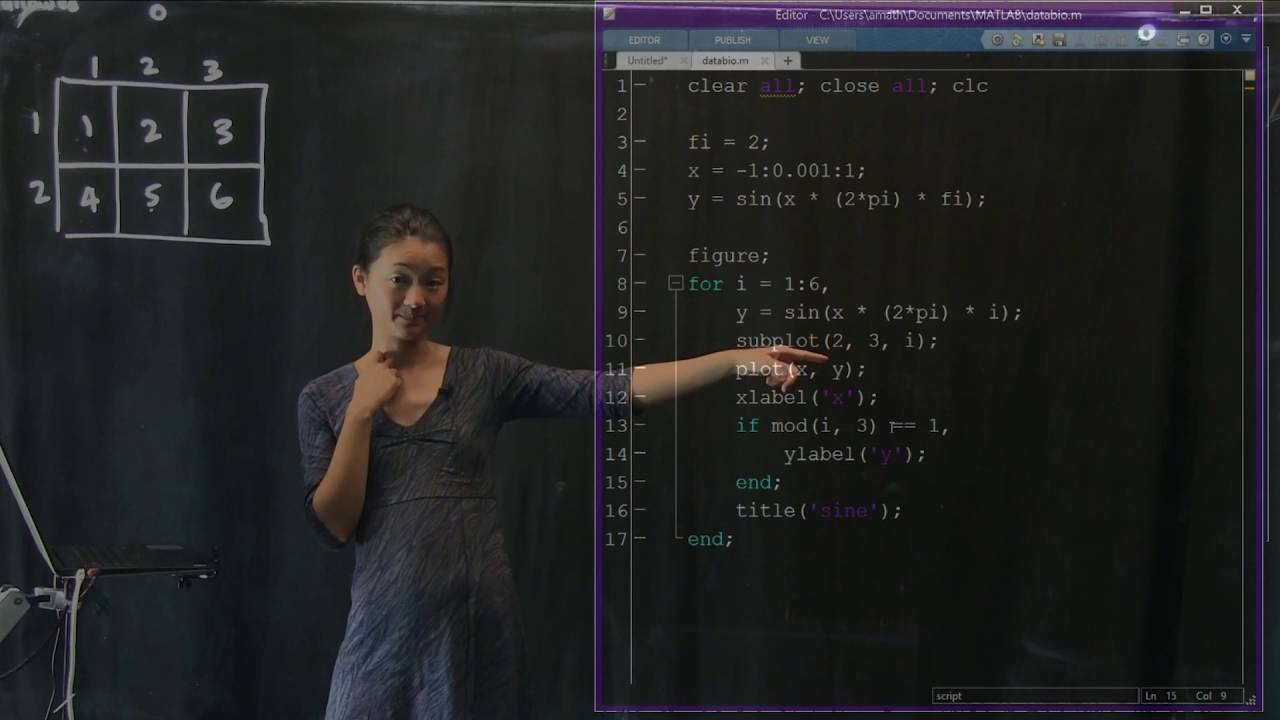
Contents
The Simplicity of Subplots
To plot multiple plots at the same time, we can use the subplot
command. This command enables us to divide our figure into a grid system, where each grid can contain a different plot. By specifying the number of rows and columns we want in our grid, we can define the layout of our plots.
Let’s start by creating a simple example. We will plot a sinusoidal function, but with different periods. We will use the subplot
command to create a grid with 2 rows and 3 columns, resulting in a total of 6 subplots. Our goal is to populate each subplot with a different plot of the sinusoidal function.
import matplotlib.pyplot as plt
import numpy as np
# Create the figure and define the subplot grid
fig = plt.figure()
grid = (2, 3)
# Populate each subplot with a different plot
for i in range(1, 7):
# Create the subplot
ax = fig.add_subplot(*grid, i)
# Define the sinusoidal function with a specific period
period = i
x = np.linspace(-1, 1, 1000)
y = np.sin(2 * np.pi * period * x)
# Plot the function
ax.plot(x, y)
# Show the figure
plt.show()
In the above code, we iterate over each subplot index using a for loop. Within each iteration, we create a new subplot by calling fig.add_subplot(*grid, i)
, where *grid
unpacks the grid dimensions and i
specifies the position of the subplot within the grid. We then define the sinusoidal function with a specific period and plot it on the subplot using ax.plot(x, y)
.
By running this code, we can see that we have successfully created six plots, each with a different period. The plots are arranged in a 2×3 grid, making it easier to compare the sinusoidal functions visually.
Customization and Fine-tuning
Now that we have learned how to create multiple plots simultaneously, let’s explore some additional customization options to enhance our plots further.
Axis Labels and Titles
To make our plots more informative, we can add axis labels and a title to each subplot. This allows us to provide context and clarify the purpose of the plot. We can do this by calling ax.set_xlabel('x')
and ax.set_ylabel('y')
to set the x-axis and y-axis labels, respectively. We can also call ax.set_title('Title')
to set the title of each subplot.
Gridlines
In certain cases, it is helpful to have gridlines in our plots, especially when comparing data. We can enable gridlines by calling ax.grid(True)
. This will display horizontal and vertical lines that make it easier to compare data points.
Marker and Line Customization
If we want to distinguish between the markers and the lines connecting them in our plots, we can customize their appearance. For example, we can change the color of the markers and lines, specify the marker size, and even modify the line width. This can be done by passing additional parameters to the ax.plot()
function.
Combining Multiple Customizations
We can combine multiple customizations to create plots that meet our specific requirements. For instance, we can modify the marker color by specifying the RGB values, adjust the line width, and even change the marker shape. By referring to the documentation for the plot
function, we can find a myriad of customization options and examples.
Conclusion
In this comprehensive guide, we have explored the power of plotting and visualizing data using subplots. We have learned how to create an array of plots in a single figure and customize each subplot to make them more informative and visually pleasing. By harnessing the full potential of the subplot
command and using various customization techniques, we can effectively analyze and interpret complex data sets.
For more information and examples on how to use the subplot
command and customize plots using Matplotlib, you can visit the official Techal website.
FAQs
Q: How can I plot multiple plots in a single figure using Python?
A: You can use the subplot
command from the Matplotlib library to create a grid of subplots within a single figure. This allows you to plot multiple plots simultaneously and compare data more efficiently.
Q: Can I customize the appearance of markers and lines in my plots?
A: Yes, you can customize the appearance of markers and lines in your plots. For example, you can change the color, size, and shape of markers, as well as adjust the line width. By referring to the documentation and using additional parameters in the plot
function, you can achieve the desired customization.
Q: How can I add axis labels and a title to each subplot in Matplotlib?
A: To add axis labels and a title to each subplot, you can use ax.set_xlabel('x')
and ax.set_ylabel('y')
to set the x-axis and y-axis labels, respectively. Additionally, you can use ax.set_title('Title')
to set the title of each subplot.
Q: Can I enable gridlines in my plots to make data comparison easier?
A: Yes, you can enable gridlines in your plots by calling ax.grid(True)
. This will display horizontal and vertical lines that help in comparing data points effectively.
Q: Where can I find more information and examples on plotting and visualizing data in Python?
A: For more information and examples on plotting and visualizing data in Python, you can visit the official Techal website. There, you can find comprehensive guides, tutorials, and resources to enhance your data visualization skills.