Welcome to the world of RESTful APIs! In this article, we will explore how to make HTTP requests using the Axios library. Whether you are a seasoned developer or just getting started, understanding how to communicate between your front-end and back-end is crucial.
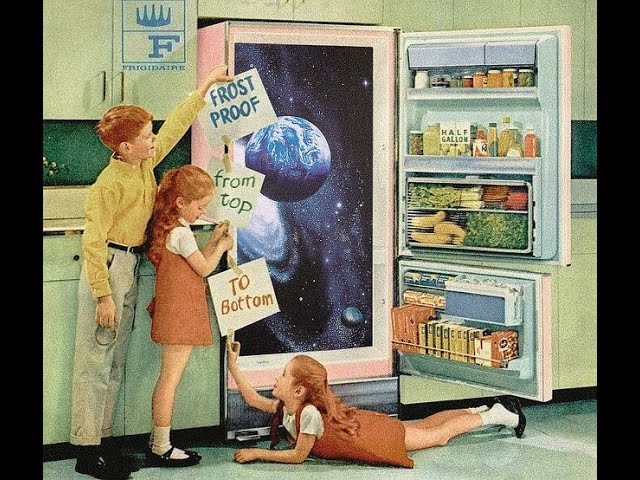
Contents
What is a REST API?
REST stands for Representational State Transfer. It is a set of architectural principles that enables communication between different systems over the internet. REST APIs use HTTP requests to send and receive data, usually in the JSON format.
Using Axios in Your Next App
To make HTTP requests from your Vue.js application, we can leverage the Axios library. Axios is a popular choice for its simplicity and flexibility. In the context of a Next.js application, we can easily set it up with the help of a Next.js module.
To get started, you can install the Next.js Axios module using npm:
npm install next-axios
Next, we need to configure the module in our project. In your next.config.js
file, you can add the Axios module as a build module:
module.exports = {
// Other configurations...
buildModules: [
'next-axios',
],
};
With the Axios module included, we can now use Axios in our Next.js app. The module provides a set of functions that start with a dollar sign, which can be used to quickly retrieve the desired information from the server’s response. For example, $get
returns only the data from the response.
Making a Test Request
Let’s make a simple test request to demonstrate how Axios works. In your Vue component, you can use the created
lifecycle hook to make the request when the component is created. Here’s an example:
export default {
data() {
return {
sections: [],
};
},
created() {
this.$get('/api/test')
.then((response) => {
this.sections = response.data;
console.log(this.sections);
})
.catch((error) => {
console.error(error);
});
},
};
In the example above, we use the $get
function to send a GET request to /api/test
, which is the endpoint we defined on the server side. We then retrieve the data from the server’s response and assign it to the sections
property in our component’s data. Finally, we log the sections
to the console.
Protecting Your API
Keep in mind that when making requests from your front-end to your back-end, you need to consider security measures such as authentication and authorization. It is essential to protect sensitive data and ensure that only authorized users can access it.
Implementing authentication and authorization mechanisms, such as user login and password protection, is crucial for securing your REST API. Additionally, you may need to handle cross-origin resource sharing (CORS) to allow requests from different domains.
Conclusion
Understanding how to make RESTful requests using Axios is a valuable skill for any developer. By following the steps outlined in this article, you can effectively communicate between your front-end and back-end, retrieve and display data, and enhance the functionality of your applications.
Remember to always put security measures in place to protect your API and its data. Stay tuned for the next article, where we will explore advanced techniques using Vuex, a powerful state management library for Vue.js. Happy coding!
FAQs
-
What is a REST API?
A REST API (Representational State Transfer) allows different systems to communicate over the internet using HTTP requests and responses. It is commonly used to send and receive data in JSON format. -
How can I make HTTP requests in my Vue.js application?
You can use the Axios library to make HTTP requests from your Vue.js application. Axios provides a simple and flexible interface for sending and handling HTTP requests. -
What is the benefit of using the Axios module in a Next.js app?
The Axios module for Next.js simplifies the setup and usage of Axios in your Next.js application. It automatically configures Axios and integrates it with the Next.js framework, making it easier to make HTTP requests from your components. -
How can I protect my REST API from unauthorized access?
To protect your REST API, you can implement authentication and authorization mechanisms. This typically involves requiring users to log in with a username and password and verifying their credentials before granting access to sensitive data or operations. -
How can I handle cross-origin resource sharing (CORS) in my REST API?
Cross-origin resource sharing (CORS) can be handled by configuring the server-side of your REST API to include the necessary headers allowing requests from specific origins. This ensures that only authorized domains can access your API and helps prevent unauthorized access to your resources.