In this tutorial, we will build an image classification script in Python using TensorFlow and Convolutional Neural Networks (CNNs). CNNs are a type of neural network that is specifically designed for processing image and audio data, or any data where patterns need to be identified.
We will use a dataset called Keras, which contains images with ten possible classifications, such as planes, trucks, and horses. We will train our neural network to recognize these images and then test it with images from the internet to see if the classification is correct.
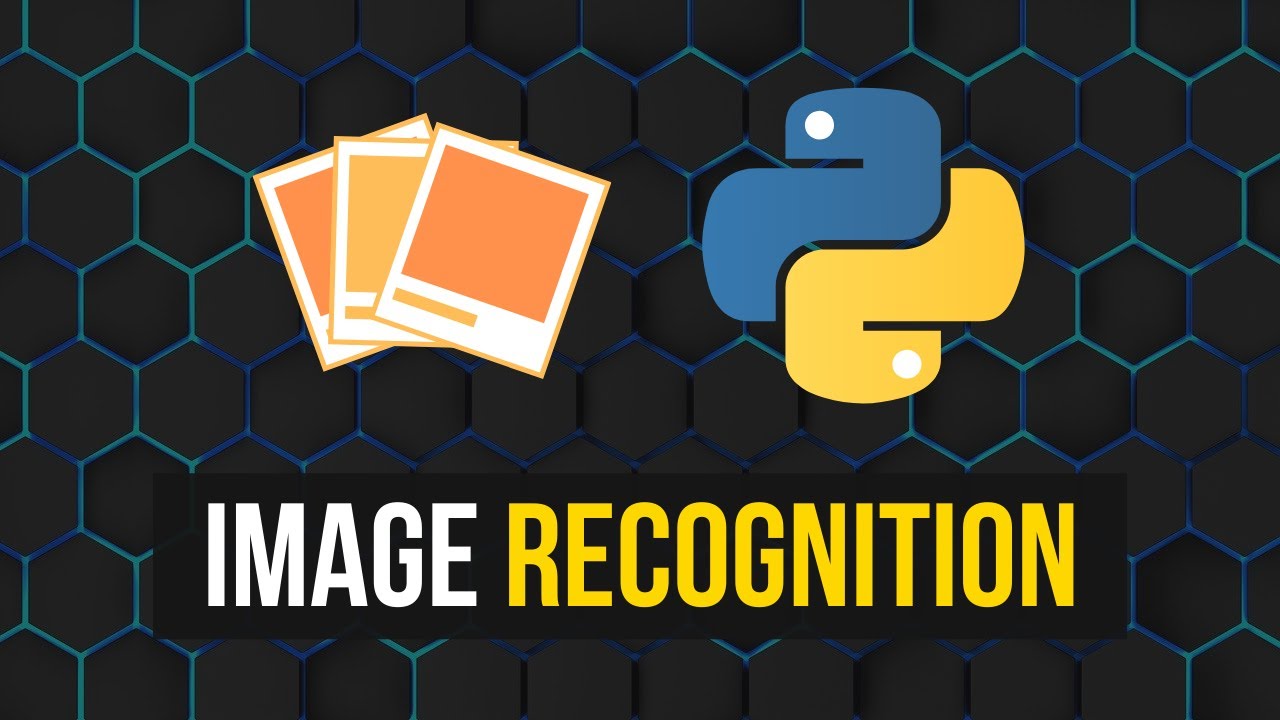
Contents
Setting Up the Environment
To get started, we need to install a few libraries. Open your terminal or command prompt and activate your virtual environment. Then, install the following libraries:
- Numpy
- Matplotlib
- Tensorflow
- OpenCV
After the installations, import the necessary libraries:
import cv2 as cv
import numpy as np
import matplotlib.pyplot as plt
from tensorflow.keras import datasets, layers, models
Loading and Preparing the Data
Next, we need to load the data from the Keras dataset and prepare it for training. The dataset includes images and their corresponding labels. We divide the data into training and testing sets.
(training_images, training_labels), (testing_images, testing_labels) = datasets.cifar10.load_data()
To normalize the data, we scale the pixel values between 0 and 1. This step is necessary to make the data more manageable for the neural network.
training_images = training_images / 255
testing_images = testing_images / 255
Visualizing the Dataset
Before training the neural network, let’s visualize some images from the dataset. We will display 16 images in a 4×4 grid to get an overview of what the dataset looks like.
class_names = ['plane', 'car', 'bird', 'cat', 'deer', 'dog', 'frog', 'horse', 'ship', 'truck']
plt.figure(figsize=(10, 10))
for i in range(16):
plt.subplot(4, 4, i+1)
plt.xticks([])
plt.yticks([])
plt.imshow(training_images[i])
plt.xlabel(class_names[training_labels[i][0]])
plt.show()
Building the Neural Network
Now, let’s define our neural network model. We will use a sequential model, which is a basic stack of layers. The model consists of convolutional layers, max pooling layers, and dense layers.
model = models.Sequential()
model.add(layers.Conv2D(32, (3, 3), activation='relu', input_shape=(32, 32, 3)))
model.add(layers.MaxPooling2D((2, 2)))
model.add(layers.Conv2D(64, (3, 3), activation='relu'))
model.add(layers.MaxPooling2D((2, 2)))
model.add(layers.Conv2D(64, (3, 3), activation='relu'))
model.add(layers.Flatten())
model.add(layers.Dense(64, activation='relu'))
model.add(layers.Dense(10, activation='softmax'))
Compiling and Training the Model
After building the model, we need to compile it by specifying the optimizer, loss function, and metrics. We will use the Adam optimizer, sparse categorical cross-entropy as the loss function, and accuracy as the metric.
model.compile(optimizer='adam',
loss=tf.keras.losses.SparseCategoricalCrossentropy(from_logits=True),
metrics=['accuracy'])
model.fit(training_images, training_labels, epochs=10, validation_data=(testing_images, testing_labels))
Evaluating the Model
Once the training is complete, we can evaluate the model’s performance on the testing dataset and print the loss and accuracy.
loss, accuracy = model.evaluate(testing_images, testing_labels)
print(f"Loss: {loss}, Accuracy: {accuracy}")
Making Predictions
Finally, we can use the trained model to make predictions on new images. We load an image, preprocess it, make a prediction, and print the predicted class name.
image = cv.imread("horse.jpg")
image = cv.cvtColor(image, cv.COLOR_BGR2RGB)
image = np.array(image) / 255
prediction = model.predict(np.array([image]))
index = np.argmax(prediction)
print(f"Prediction: {class_names[index]}")
Conclusion
In this tutorial, we have built an image classification script using convolutional neural networks in Python. We loaded and prepared the data, built the neural network model, trained and evaluated the model, and made predictions on new images.
Using this script, you can classify images with high accuracy and recognize objects in real-world scenarios. Feel free to experiment with different datasets and improve the model’s performance. Happy coding!
FAQs
Q: Can I train the model on my own dataset?
Yes, you can train the model on your own dataset. Replace the training_images
and training_labels
variables with your custom dataset, ensuring that the images are in RGB format and scaled between 0 and 1.
Q: How can I improve the model’s accuracy?
You can experiment with different architectures, hyperparameters, and data augmentation techniques to improve the model’s accuracy. Consider increasing the number of layers, using larger or more complex networks, adjusting the learning rate, or adding regularization techniques.
Q: What other applications can this model be used for?
This model can be applied to various image classification tasks, such as detecting objects in images, recognizing handwritten digits, identifying facial expressions, and more. It can be customized to suit your specific application by training it on a relevant dataset.