Welcome to another exciting episode of game programming! In this episode, we will delve into the fascinating world of offsets and tile rendering. By the end, you will have a firm grasp on how to dynamically adjust the position of tiles on the map, allowing for smooth movement of in-game elements.
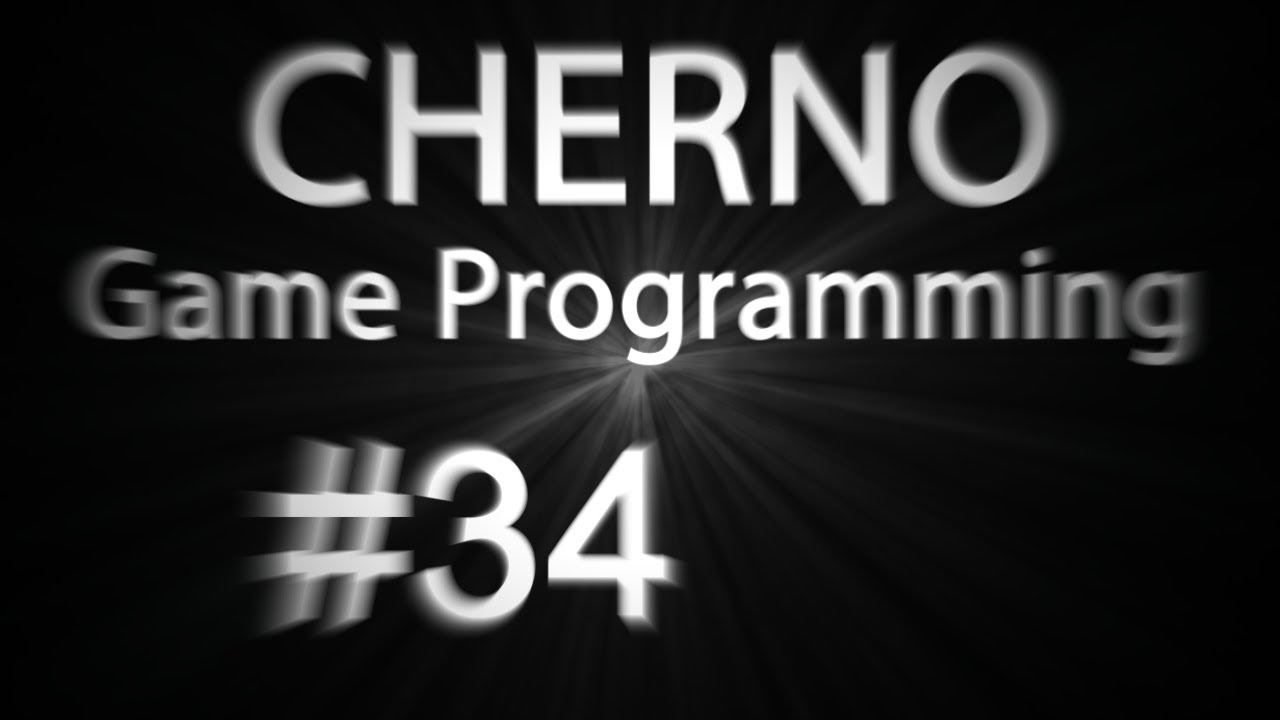
Contents
Understanding Offsets
Before we dive into the details, let’s quickly recap what offsets are. In game development, offsets are values that allow us to reposition objects on the screen. When the player moves, the map needs to adjust accordingly to create the illusion of movement. The offset values determine how much we need to shift the map to keep the player centered.
Introducing the Set Offset Method
To begin, we need to create a method that will handle the setting of offsets. In the Screen
class, below the renderTile
method, we will define two variables: Xoffset
and Yoffset
. These variables will store the offset values.
public int Xoffset;
public int Yoffset;
Now, let’s create a public method called setOffset
within the Screen
class. This method will accept two integer parameters: Xoffset
and Yoffset
. Within this method, we will assign the input values to the corresponding class variables.
public void setOffset(int Xoffset, int Yoffset) {
this.Xoffset = Xoffset;
this.Yoffset = Yoffset;
}
In simpler terms, when we call the setOffset
method, we provide two integer values for Xoffset
and Yoffset
. These values are then stored in the class variables, allowing us to access and use them in other methods.
Applying Offsets to Tile Rendering
Now comes the exciting part – applying the offsets to our tile rendering process. Previously, we were rendering tiles based on their absolute position on the map. However, this approach doesn’t account for the player’s movement, resulting in a static map where the tiles do not adjust when the player moves.
To rectify this, we need to modify the renderTile
method in the Screen
class. Instead of directly setting the XP
and YP
variables, we will add the offsets to these variables to dynamically adjust the tile positions.
For example, let’s say the player moves 200 pixels to the right. We would want to ensure that the map shifts left by 200 pixels. To achieve this, we modify the code as follows:
XP = XP - Xoffset;
YP = YP - Yoffset;
These lines of code subtract the offset values from the original tile positions. By doing this, we effectively shift the entire map in the opposite direction of the player’s movement, creating a smooth and immersive experience.
Plugging in the Offsets
To make use of our new offset functionality, we need to call the setOffset
method at the appropriate time. In the Level
class, within the rendering loop, we can do this right before rendering the tiles. Simply add the following line of code:
screen.setOffset(Xscroll, Yscroll);
This code calls the setOffset
method of the Screen
class and passes in the current scroll values of the player. These scroll values represent the player’s location on the map and will be used to calculate the offsets.
Conclusion
Congratulations! You are now equipped with the knowledge and skills to implement offsets and dynamically adjust the position of tiles in your game. By using offsets, you can create a seamless and immersive gaming experience where the map seamlessly moves with the player.
Stay tuned for the next episode, where we will explore the exciting world of rendering tiles onto the screen. Until then, happy coding!
FAQs
Q: What are offsets in game development?
A: Offsets are values used to reposition objects on the screen. They are particularly useful for adjusting the map when the player moves.
Q: How do I set offsets in my game?
A: To set offsets, you can create a setOffset
method in your code that accepts the desired offset values. These values can then be applied in the rendering process to dynamically adjust the positions of objects.
Q: Can I use offsets to create smooth movement in my game?
A: Yes! By properly applying offsets to the rendering process, you can achieve smooth movement effects in your game. Offsets allow you to dynamically reposition objects based on player input, creating an immersive gaming experience.
Reference:
To learn more about game programming and explore further exciting topics, visit Techal.