Welcome to Episode 47 of the Game Programming series! In the previous episode, we wrapped up the King Jonas sprite, and today we’re taking a different approach to rendering it. Currently, we are using a quad render technique, dividing the player into four quarters and rendering them separately.
This method works well and the frames per second (FPS) drop is minimal. However, we can optimize the rendering process further by rendering the player sprite in one go.
To understand the implementation, let’s dive into the code. Currently, our player sprites are 16 by 16 pixels. We want to increase their size to 32 by 32 pixels to encapsulate every single sprite in one rendering.
public static Sprite player = new Sprite(32, /* sprite sheet location */);
By doubling the sprite size, we need to adjust the rendering coordinates. For example, if previously the player sprite was located at the tenth sprite position (16 pixels downwards), with 32-pixel size sprites, we will now target the fifth sprite position (32 pixels downwards).
It is important to note that the size multiplication factor affects the starting position of the sprite on the y-axis. To compensate for this, we halve the original position count.
To visualize this concept, imagine multiplying 16 by 10 (the original sprite position), resulting in 160 pixels. With 32-pixel size sprites, we multiply 32 by 5 to reach the same pixel count.
To implement the changes, update the renderPlayer
method to use the new sprite size:
renderPlayer(32, 32, ...);
With this simple adjustment, we have successfully rendered the player sprite using the enhanced 32-size sprites without any noticeable decrease in performance.
It is worth mentioning that collision detection becomes slightly more advanced with 32-size rendering. However, we will cover that topic in a future episode.
And there you have it! We hope you enjoyed this episode of game programming. If you found it helpful, don’t forget to hit the like button below the video. Stay tuned for our next episode, where we will delve into sprite animation.
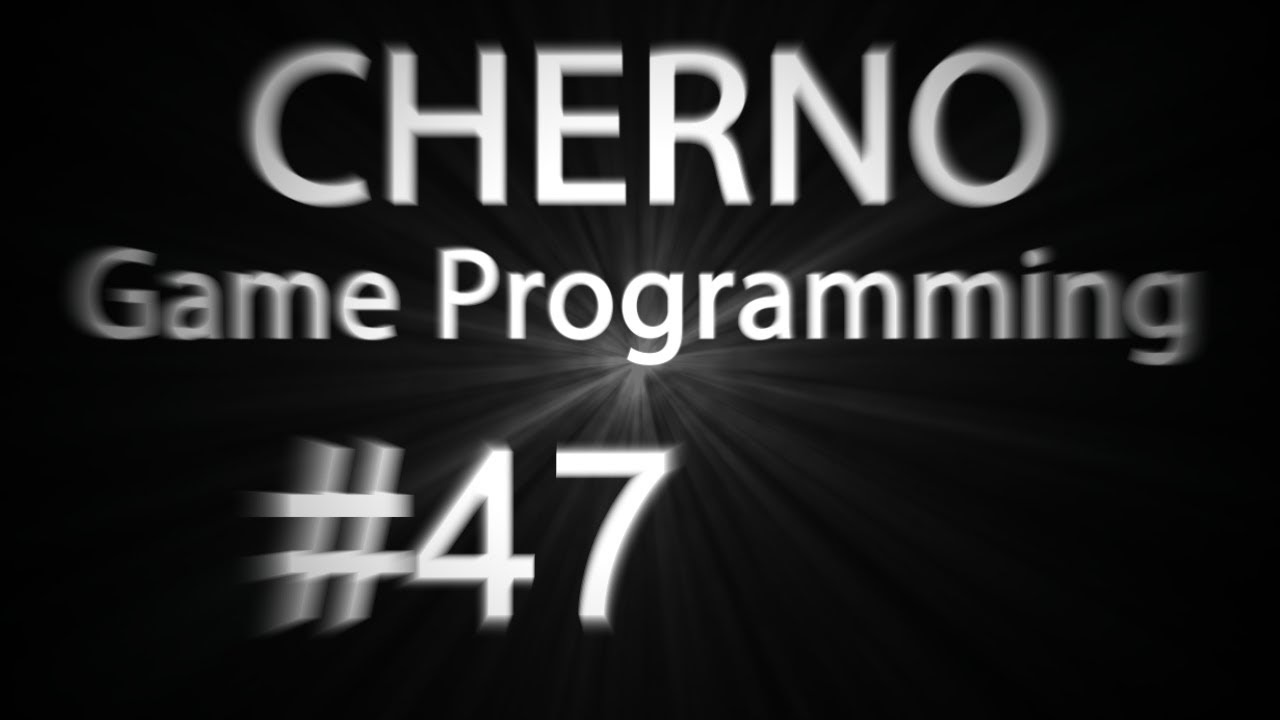
Contents
FAQs
Q: How does rendering the player sprite in one go improve performance?
A: Rendering the player sprite in one go minimizes the rendering operations required by the computer, resulting in optimized performance.
Q: Why do we need to adjust the starting position of the sprite when using 32-pixel size sprites?
A: When increasing the sprite size, the starting position on the y-axis needs to be adjusted to maintain accurate rendering.
Q: Will increasing the sprite size affect collision detection?
A: Yes, collision detection becomes slightly more complex when using 32-size rendering, but we will cover that topic in a future episode.
Conclusion
In this episode, we explored the benefits of rendering player sprites using 32-size sprites. By making this simple adjustment, we were able to enhance performance without sacrificing visual quality. Stay tuned for our next episode, where we will delve into sprite animation. Thank you for watching, and see you next time!