Do you want to create a home automation project using the ESP32? The ESP32 comes with an inbuilt capacitive touch sensor that allows you to control relays without the need for external touch sensors. In this tutorial, we will show you how to use the ESP32’s touch sensor to control relays, and even discuss how to control multiple relays using both the built-in and external touch sensors.
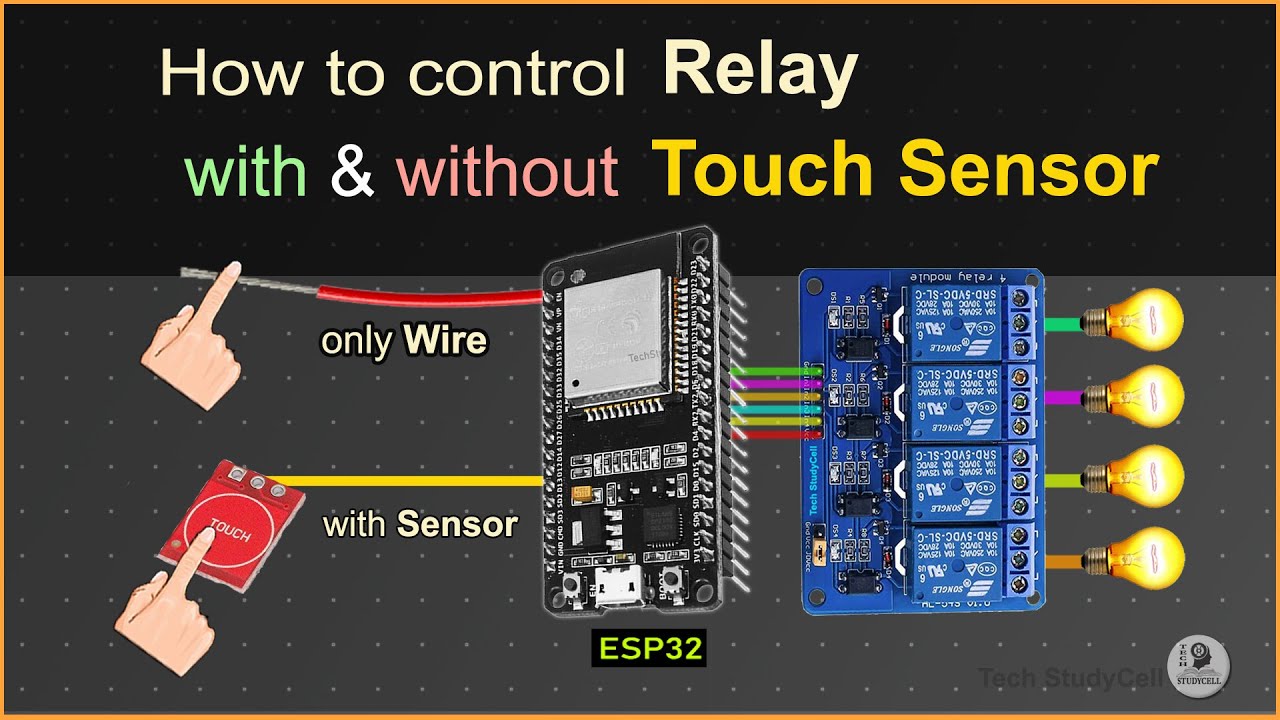
Controlling a Single Relay
Let’s start by controlling a single relay using the ESP32’s inbuilt touch sensor. In this setup, the touch pin is connected to GPIO 27, and the relay is connected to the ESP32. When you touch the wire connected to GPIO 27, the relay will turn on, and when you remove your hand, the relay will turn off.
Here is a simple circuit diagram for this setup:
To write the code, you can use any IDE of your choice, such as Arduino IDE. Here is a sample code snippet:
int relayPin = 13; // the GPIO pin connected to the relay
int touchPin = T7; // the touch pin connected to GPIO 27
void setup() {
pinMode(relayPin, OUTPUT);
pinMode(touchPin, INPUT);
}
void loop() {
int touchValue = touchRead(touchPin);
if(touchValue < 40){
digitalWrite(relayPin, HIGH);
}else{
digitalWrite(relayPin, LOW);
}
}
Upload this code to your ESP32, and you should be able to control the relay by touching the wire connected to GPIO 27.
Controlling Multiple Relays
Now, let’s explore how to control multiple relays using both the inbuilt and external touch sensors. While you can control multiple relays using the inbuilt touch sensor, there is a limitation. When you try to control one relay, another relay may automatically turn on. To overcome this limitation, we recommend using an external touch sensor.
Here is a circuit diagram for controlling four relays without using any external touch sensors:
To control multiple relays, you can refer to the circuit diagram above. However, be aware that using the inbuilt touch sensor alone may result in unintended relay activation. To resolve this issue, we recommend using an external touch sensor.
To program this setup, you can modify an example code provided by the ESP32 touch library to control multiple relays. Here’s an example code snippet:
int relayPins[] = {2, 4, 5, 6}; // the GPIO pins connected to the relays
int touchPins[] = {T0, T3, T6, T9}; // the touch pins connected to the external touch sensors
void setup() {
for(int i = 0; i < 4; i++){
pinMode(relayPins[i], OUTPUT);
pinMode(touchPins[i], INPUT);
}
}
void loop() {
for(int i = 0; i < 4; i++){
int touchValue = touchRead(touchPins[i]);
if(touchValue < 40){
digitalWrite(relayPins[i], HIGH);
}else{
digitalWrite(relayPins[i], LOW);
}
}
}
Upload this code to your ESP32, and you should be able to control multiple relays using both the inbuilt and external touch sensors.
Conclusion
By using the ESP32’s inbuilt capacitive touch sensor, you can easily control relays in your home automation projects. Whether you want to control a single relay or multiple relays, the ESP32 offers a simple and effective solution. Remember to consider the limitations of using only the inbuilt touch sensor and to use an external touch sensor if necessary.
We hope this guide has been helpful in getting you started with the ESP32 touch sensor and relay control. For more informative content about the ever-evolving world of technology, stay tuned to Techal.
FAQs
Q: Can I control relays using only the ESP32’s inbuilt touch sensor?
A: Yes, you can control relays using the ESP32’s inbuilt touch sensor. However, be aware that there may be limitations, such as unintended relay activation.
Q: How can I control multiple relays using the inbuilt touch sensor?
A: While it is possible to control multiple relays using the inbuilt touch sensor, be aware that there may be issues with unintended relay activation. To avoid this, we recommend using an external touch sensor.
Q: Can I use the ESP32 touch sensor with other home automation projects?
A: Yes, you can easily modify the code provided in this tutorial to add the touch sensor to any other home automation project.
Q: Where can I find the circuit diagrams and code mentioned in this tutorial?
A: You can find the circuit diagrams and code in the related article on Techal.
Conclusion
In this tutorial, we explored how to control relays using the ESP32’s inbuilt capacitive touch sensor. We discussed how to control a single relay and how to control multiple relays using both inbuilt and external touch sensors. Remember to consider the limitations of using only the inbuilt touch sensor and to use an external touch sensor if necessary.
We hope this tutorial has empowered you with the knowledge to incorporate touch sensor control in your home automation projects. For more informative content about the ever-evolving world of technology, stay tuned to Techal.