In the previous video, we discovered a limitation in our code – the generated results were always converging to the same value. To address this, we need to introduce more chaos into our calculations. We want small changes in the input to yield detectable differences in the output. Chaos will enable us to identify and address race conditions effectively.
To achieve this, we will use a range of sine and cosine values. We will start by converting our values to doubles and using a sine and cosine range between 0 and π. This will provide more randomness in our calculations.
To introduce greater chaos, we will modify the values by slicing out some digits and using them in the next iteration. First, we will multiply the values by a large number (e.g., 10 million), take the absolute value, and then perform modulo operations to obtain a value between 0 and 99,000. These modifications will inject more entropy into our calculations.
To measure the impact of these changes, we will record the time taken for each chunk and each thread within a chunk using a chili timer. This will give us insights into the workload distribution and the time spent working by each thread.
All the timing data will be stored in a struct called “chunk timing info.” We will loop through each chunk and each thread within a chunk to retrieve the respective work time, idle time, and number of heavy items processed. This information will be pushed back into a vector called “timings.”
Once the processing is complete, we will output the recorded timings to a CSV file. We will first output the headers, including the work time, idle time, and number of heavy items processed per thread. For each chunk, we will output the total chunk time and the total number of heavy items. This will provide a comprehensive overview of our multithreading performance.
At last, we will have a CSV file that contains all the necessary data to analyze and optimize our multithreading code. This will enable us to make informed decisions to improve performance and ensure a balanced workload distribution.
With these changes, we have successfully unleashed chaos in our calculations and implemented a robust measurement system to analyze the performance of our multithreading algorithm. The combination of chaos and thorough measurement will empower us to make data-driven decisions and optimize our code effectively.
Stay tuned for more exciting developments as we continue to explore the world of multithreading in C++.
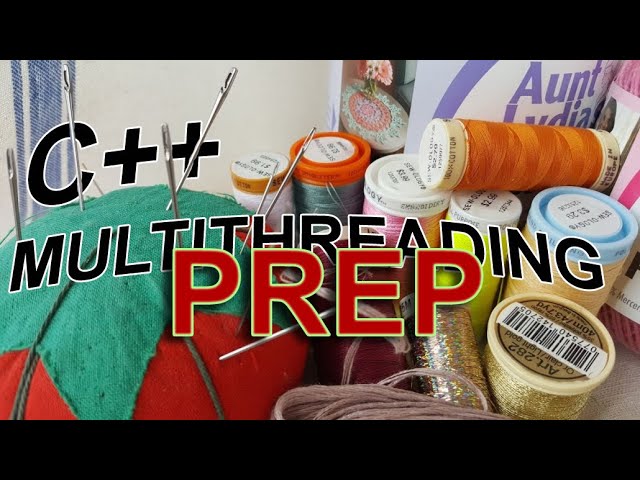
Contents
FAQs
-
Why is injecting chaos important in multithreading?
Injecting chaos into multithreading algorithms is crucial because it allows us to identify and address race conditions effectively. Chaos introduces randomness and variability in calculations, making it easier to detect discrepancies caused by race conditions. -
How can we measure the performance of multithreading algorithms?
To measure the performance of multithreading algorithms, we can use timing tools such as the Chili Timer. By measuring the time taken by each thread and tracking the workload distribution, we can gain insights into the efficiency and effectiveness of our multithreading algorithm. -
How can we balance the workload distribution in multithreading?
To balance the workload distribution in multithreading, we can allocate tasks evenly across threads. By ensuring that each thread receives an equal amount of work, we can prevent bottlenecks and optimize overall performance.
Conclusion
With chaos injected into our multithreading algorithm and a robust measurement system in place, we have laid the foundation for optimizing our code. By carefully analyzing the performance data, we can fine-tune the workload distribution and address any potential race conditions. Stay tuned for more updates as we continue to explore the exciting world of multithreading in C++.