Have you ever wanted to build a machine learning application with a graphical user interface? Look no further than Gradio. In this article, we’ll explore how Gradio can be used to quickly and easily develop a machine learning application using a handwritten digit recognition as an example.
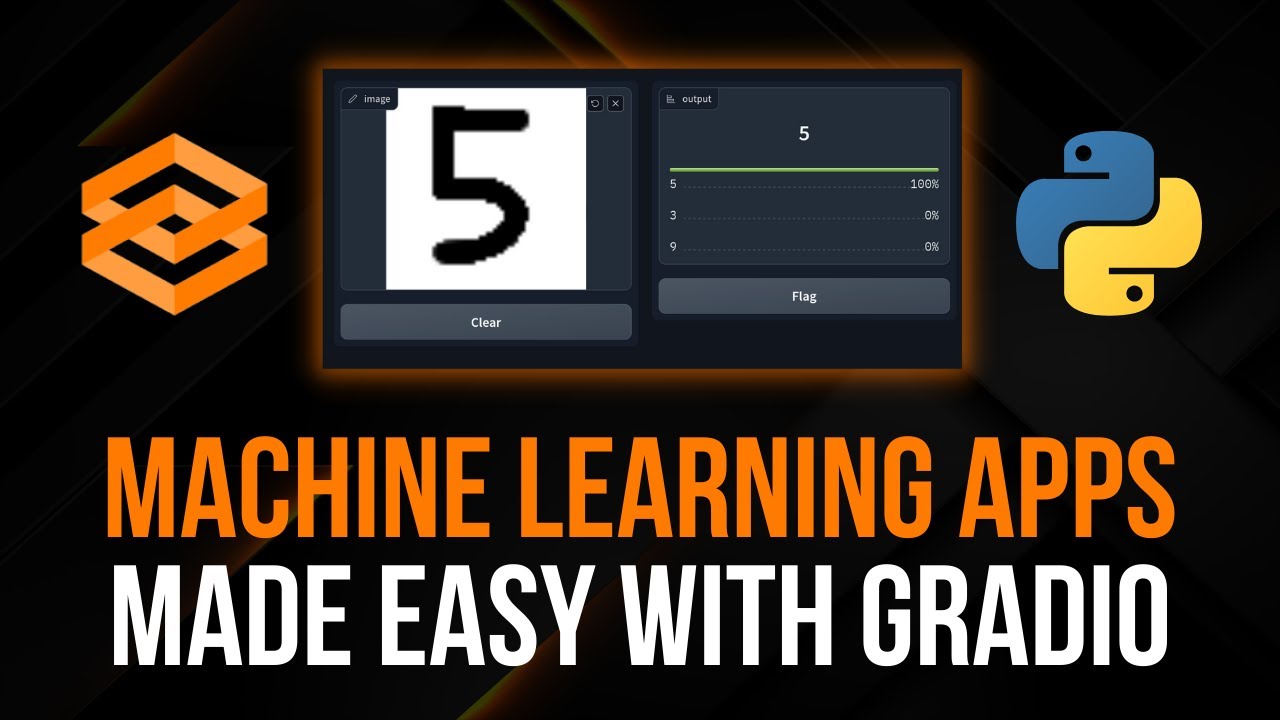
Contents
Getting Started with Gradio and Handwritten Digit Recognition
Before we dive into building the application, let’s take a moment to understand what we’ll be creating. The application will consist of a canvas on the left side where users can draw a digit, and on the right side, the model will provide predictions for the drawn digit along with the likelihood of other possible digits.
To begin building our application, there are two packages we’ll need to install: Gradio and a machine learning framework of your choice (e.g., TensorFlow, PyTorch, or Scikit-learn). Open up your command line or terminal and use the following commands to install the required packages:
pip install gradio
pip install tensorflow
Once the installations are complete, we can start creating our application. We’ll split the process into two parts: training the model and building the application. Let’s start by training the model.
Training the Handwritten Digit Recognition Model
In order to train our model, we’ll use the MNIST dataset, which consists of handwritten digit images. We can load this dataset directly from TensorFlow using the following code:
from tensorflow.keras.datasets import mnist
(train_images, train_labels), (test_images, test_labels) = mnist.load_data()
Next, we’ll normalize the images by dividing the pixel values by 255, which will scale them to a range of 0 to 1:
train_images = train_images.reshape(60000, 28, 28, 1).astype('float32') / 255
test_images = test_images.reshape(10000, 28, 28, 1).astype('float32') / 255
We’ll also convert the labels into categorical variables using one-hot encoding:
from tensorflow.keras.utils import to_categorical
train_labels = to_categorical(train_labels)
test_labels = to_categorical(test_labels)
Now, it’s time to define our neural network architecture. We’ll build a simple architecture consisting of convolutional and dense layers:
from tensorflow.keras import models, layers
model = models.Sequential()
model.add(layers.Conv2D(32, (3, 3), activation='relu', input_shape=(28, 28, 1)))
model.add(layers.MaxPooling2D((2, 2)))
model.add(layers.Conv2D(64, (3, 3), activation='relu'))
model.add(layers.MaxPooling2D((2, 2)))
model.add(layers.Conv2D(64, (3, 3), activation='relu'))
model.add(layers.Flatten())
model.add(layers.Dense(64, activation='relu'))
model.add(layers.Dense(10, activation='softmax'))
Once our model is defined, we’ll compile it with the appropriate optimizer, loss function, and metric:
model.compile(optimizer='adam',
loss='categorical_crossentropy',
metrics=['accuracy'])
Now, it’s time to train the model using the training data:
model.fit(train_images, train_labels, epochs=5, batch_size=64, validation_split=0.1)
Once the training is complete, we can save the model for future use:
model.save('model.h5')
Building the Gradio Application
With the model trained and saved, we can now build the Gradio application. Create a new Python file, import the necessary packages, and load the model:
import gradio as gr
import tensorflow as tf
model = tf.keras.models.load_model('model.h5')
Next, we’ll define a function that takes an image as input and returns the predictions:
def recognize_digit(image):
if image is not None:
image = image.reshape(1, 28, 28, 1).astype('float32') / 255
prediction = model.predict(image)
probabilities = {str(i): float(prediction[0][i]) for i in range(10)}
return probabilities
else:
return ""
Now, we can create the Gradio interface by specifying the input and output components:
iface = gr.Interface(fn=recognize_digit, inputs="image", outputs="label",
title="Handwritten Digit Recognition",
description="Draw a digit and see the model's predictions.")
iface.launch()
When you run the code, the Gradio interface will launch, allowing you to draw digits on the canvas and see the model’s predictions. You can customize the interface by adjusting various parameters such as the number of classes to display and the source (canvas or image upload).
FAQs
Q: Can I use a different machine learning framework?
Yes, you can use a different machine learning framework such as PyTorch or Scikit-learn. Just make sure to modify the code accordingly.
Q: How can I improve the model’s accuracy?
You can try experimenting with different architectural configurations, increasing the number of training epochs, or using data augmentation techniques to improve the model’s accuracy.
Q: Can I resize the canvas or change its shape?
Yes, you can resize the canvas or change its shape by modifying the parameters in the Gradio interface.
Conclusion
In this article, we’ve explored how to build a machine learning application with a graphical user interface using Gradio. By combining Gradio with a trained model, you can create interactive applications that allow users to provide input and receive real-time predictions. Experiment with different models and datasets to create unique and engaging applications.
To learn more about technology and stay up to date with the latest trends, visit Techal. Happy coding!