Welcome back! In this lesson, we will cover line clipping and scaling in the advanced programming tutorial series.
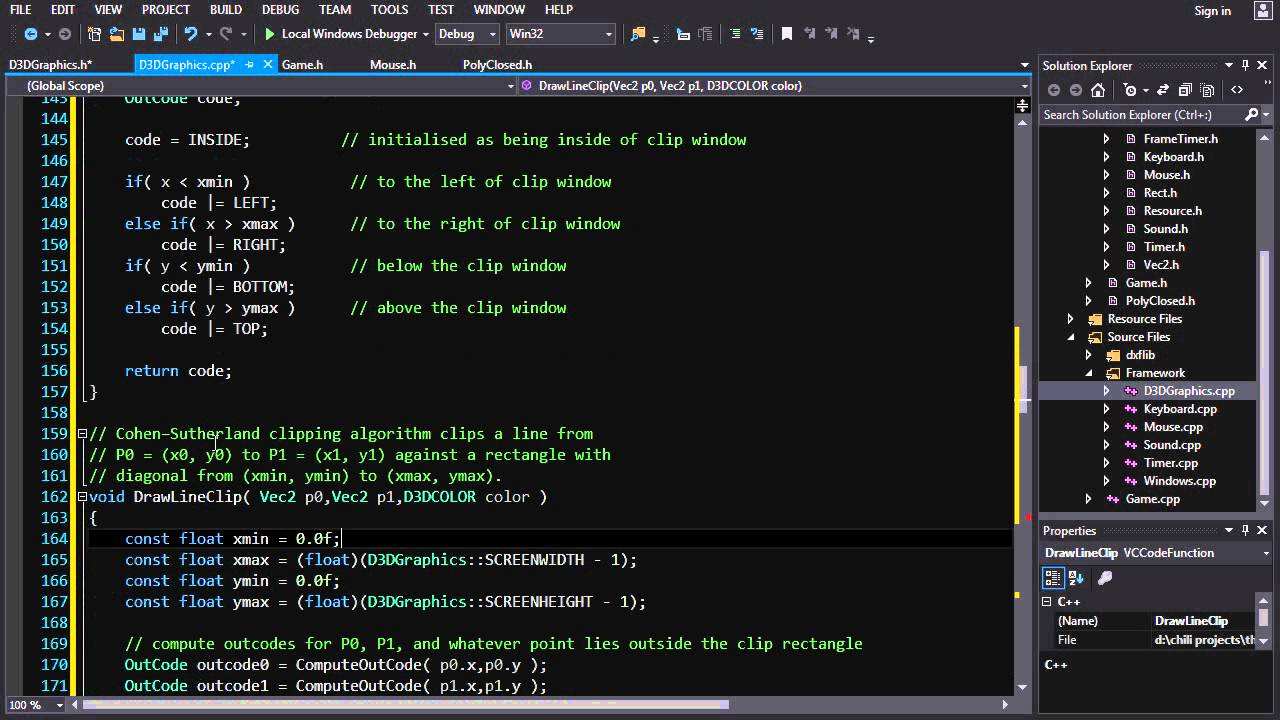
Introducing Line Clipping
Line clipping is the process of determining the portion of a line that is visible within the boundaries of a viewport. In our game, we will have lines running off the screen, and we need to ensure that only the visible parts are drawn.
To understand line clipping, let’s first discuss the basic premise of our game. You control a ship that flies around using semi-accurate Newtonian physics. The ship has a shield that allows you to ricochet off walls, but it consumes energy. The objective is to reach the finish line within a time trial. There will also be environmental hazards, such as black holes and obstacles, to make the game more challenging and interesting.
Now, let’s dive into line clipping. In our game, the screen size will be larger than a single viewport. Therefore, we need to ensure that lines are clipped correctly to fit within the viewport.
How to Clip a Line
Clipping a line using a pixel-by-pixel approach is inefficient and unnecessary. Instead, we can modify the line’s geometry to ensure that it falls within the viewport. This approach is more efficient and provides the same visual result.
To achieve this, we can use the Cohen-Sutherland algorithm, which divides the region around the viewport into eight sections. Depending on the start and end points’ characterization, the algorithm calculates intersections and revises the end points until the line is entirely in the viewport or entirely out. By modifying the end points, we can determine which pixels need to be drawn.
Implementing the Cohen-Sutherland algorithm manually can involve complex calculations and handling different cases. To simplify this process, we can find existing code or libraries that provide a pre-built implementation. One popular resource is Wikipedia, which provides a C++ implementation of the Cohen-Sutherland algorithm.
To incorporate this code into our program, we need to make some adjustments. We’ll create a new function, drawLineClip
, which will handle line clipping. This function will call the code we found on Wikipedia.
With this new code, our lines will be correctly clipped to fit within the viewport, ensuring a smooth and accurate display.
Scaling for Visual Enhancement
In addition to line clipping, we can implement scaling to enhance our game’s visual appeal. Scaling allows us to resize our objects to create different effects.
To add scaling to our game, we will integrate the scroll wheel of the mouse. We will modify our existing Mouse class to include event-based scrolling functionality, similar to what we did for the keyboard.
Once the mouse event handling is in place, we can use the scroll wheel events to control the scaling factor. When the wheel is scrolled up, we increase the scale factor, and when it is scrolled down, we decrease it. This interaction will allow users to scale and resize objects within the game, adding an additional layer of interactivity.
Conclusion
In this lesson, we explored the concepts of line clipping and scaling. Line clipping ensures that only the visible portion of a line is drawn within the viewport, while scaling allows us to resize objects dynamically for visual enhancement.
In the next lesson, we will dive into matrices and discuss their role in game development. Stay tuned for more exciting content from Techal!
FAQs
-
What is line clipping?
Line clipping is the process of determining the portion of a line that is visible within the boundaries of a viewport. -
How can I clip lines efficiently?
Rather than checking each pixel individually, you can modify the line’s geometry to fit within the viewport using the Cohen-Sutherland algorithm or a similar method. -
How can I scale objects in my game?
You can implement scaling by using events from the mouse scroll wheel. When the wheel is scrolled up, increase the scale factor, and when it is scrolled down, decrease it. -
How can I learn more about matrices in game development?
Matrix transformations are an essential concept in game development. In the next lesson, we will explore matrices in more detail, so stay tuned!
Remember to visit the Techal website for more informative articles and tutorials.